How to output unicode characters in C/C++
10,488
Most of those characters take more than a byte to encode, but std::cout
's currently imbued locale will only output ASCII characters. For that reason you're probably seeing a lot of weird symbols or question marks in the output stream. You should imbue std::wcout
with a locale that uses UTF-8 since these characters are not supported by ASCII:
// <locale> is required for this code.
std::locale::global(std::locale("en_US.utf8"));
std::wcout.imbue(std::locale());
std::wstring s = L"šđč枊ĐČĆŽ";
std::wcout << s;
For Windows systems you will need the following code:
#include <iostream>
#include <string>
#include <fcntl.h>
#include <io.h>
int main()
{
_setmode(_fileno(stdout), _O_WTEXT);
std::wstring s = L"šđč枊ĐČĆŽ";
std::wcout << s;
return 0;
}
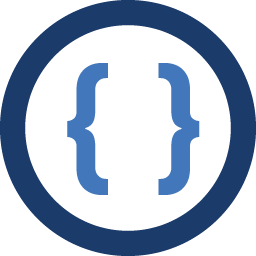
Author by
Admin
Updated on June 14, 2022Comments
-
Admin almost 2 years
I have problems with outputing unicode characters in Windows console. I am using Windows XP and Code Blocks 12.11 with mingw32-g++ compiler.
What is the proper way to output unicode characters in Windows console with C or C++?
This is my C++ code:
#include <iostream> #include <string> using namespace std; int main() { cout << "šđč枊ĐČĆŽ" << endl; // doesn't work string s = "šđč枊ĐČĆŽ"; cout << s << endl; // doesn't work return 0; }
Thanks in advance. :)