How to over-write the property in Ant?
Solution 1
Depending on how you want to use the modified property, you can use macrodef
s.
For example, instead of writing the following:
<target name="foo">
<echo message="${my_property}"/>
</target>
and not being able to call ant foo
with another message, you could write:
<macrodef name="myecho">
<attribute name="msg"/>
<sequential>
<echo message="@{msg}"/>
</sequential>
</macrodef>
<target name="foo">
<myecho msg="${my_property}"/>
<property name="my_property2" value="..."/>
<myecho msg="${my_property2}"/>
</target>
Solution 2
ant-contrib's Variable
task can do this:
<property name="x" value="6"/>
<echo>${x}</echo> <!-- will print 6 -->
<var name="x" unset="true"/>
<property name="x" value="12"/>
<echo>${x}</echo> <!-- will print 12 -->
Not recommended, though, it can lead to weird side-effects if parts of your Ant scripts assume immutable property values, and other parts break this assumption.
Solution 3
For the sake of justice, there is a hack that allows to alter ant's immutable properties without any additional libs (since java 6):
<scriptdef name="propertyreset" language="javascript"
description="Allows to assign @{property} new value">
<attribute name="name"/>
<attribute name="value"/>
project.setProperty(attributes.get("name"), attributes.get("value"));
</scriptdef>
Usage:
<property name="x" value="10"/>
<propertyreset name="x" value="11"/>
<echo>${x}</echo> <!-- will print 11 -->
As others mentioned, this should be used with care after all canonical approaches proved not to fit.
Solution 4
Properties are immutable in ant.
You may be interested in ant-contrib's var
task.
<var name="my_var" value="${my_property}" />
<echo>Addressed in the same way: ${my_var} and ${my_property}</echo>
Solution 5
Since Ant 1.8, you can use the "local" task to change the value of a property within a target. Note that this does NOT change the value of the global property with the same name but it is a way to solve some problems.
See
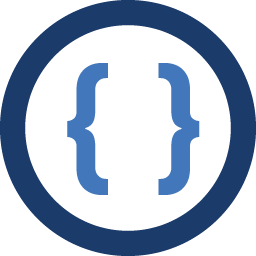
Admin
Updated on August 23, 2020Comments
-
Admin over 3 years
Is there a way to re-assign the value for the Ant
property
task? Or is there another task available for that purpose? -
Andreas Kraft over 14 yearsThe var task is especially nice for "local variables", e.g. in for loops (also a task from the excellent ant-contrib). One drawback, though, is that the var task doesn't support the "location" attribute.
-
kailash gaur over 10 yearsit's very helpful if you give one example and explain more.