How to override a global style (that doesn't have an x:Key), or alternatively apply a named style to all type-targeted controls?
Solution 1
Try this:
<Style TargetType="{x:Type StackPanel}" BasedOn="{StaticResource {x:Type StackPanel}}">
<!-- ... -->
</Style>
I have declared my base styles in a ResourceDictionary in App.xaml, if i override them in a specific window like this, it usually works.
Solution 2
Somewhere in global resource dictionary you define a base style with a key. This base style is targeted to a type which is base for all types for which you intend to apply that style. Then you define styles which target the types that you want and are based on the above mentioned base style.
<Style
x:Key="upDownBaseStyle"
TargetType="{x:Type Control}">
<Setter
Property="Margin"
Value="2" />
<Setter
Property="HorizontalAlignment"
Value="Stretch" />
<Setter
Property="VerticalAlignment"
Value="Center" />
</Style>
<Style
TargetType="{x:Type xceed:IntegerUpDown}"
BasedOn="{StaticResource upDownBaseStyle}">
</Style>
<Style
TargetType="{x:Type xceed:DoubleUpDown}"
BasedOn="{StaticResource upDownBaseStyle}">
</Style>
Now the two last styles are applied to all the IntegerUpDown and DoubleUpDown controls within your application without any mention of a key.
So the base rule: the base style must have the key to refer to it, and the derived styles may not and therefore they can be applied without any keys - only by target types.
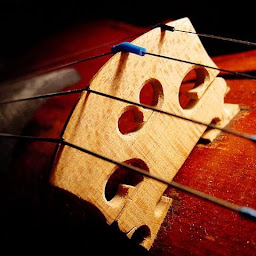
Shimmy Weitzhandler
Updated on June 06, 2022Comments
-
Shimmy Weitzhandler almost 2 years
I declared a style that I want to apply to ALL the buttons in the project, the style resides in the ResourceDictionary:
<Style TargetType="StackPanel"> <Setter Property="Orientation" Value="Horizontal" /> <Setter Property="VerticalAlignment" Value="Center"/> <Setter Property="HorizontalAlignment" Value="Center"/> </Style>
Now, in some window, I want to inherit from this style but add a value:
<Style TargetType="StackPanel"> <Setter Property="Margin" Value="5"/> </Style>
The problem is that it doesn't inherit from the global style, in order to inherit I have to assign a key to the global style:
<Style TargetType="StackPanel" x:Key="StackPanelStyle" />
And then in the window's XAML inherit (or/and override - optional) it:
<Style TargetType="StackPanel" BasedOn="StackPanelStyle" />
The problem is that if you assign a key, it's not global and you have to call it on each window/scope.
A solution to my question should be one of the two (is there anything else I am missing out?):
- Have a global style with a key, that is automatically applied to all its targeted controls in the entire application.
- A way to refer to the ResourceDictionary-level unnamed style without and override it.
I thought about redeclaring the styles near the named styles (in the ResourceDictionary) which actually works:
<!--In the ResourceDictionary--> <Style x:Key="StackPanelStyle" TargetType="StackPanel"> <Setter Property="Orientation" Value="Horizontal" /> <Setter Property="VerticalAlignment" Value="Center"/> <Setter Property="HorizontalAlignment" Value="Center"/> </Style> <!--In the app.xaml--> <Style TargetType="StackPanel" BasedOn="{StaticResource StackPanelStyle}"/> <!--In the window/page scope--> <Style TargetType="StackPanel" BasedOn="{StaticResource StackPanelStyle}"/
But I am looking for better stuff than stupidly redeclare all the styles.