How to parse HTTP responses in C?
Solution 1
http-parser is a simple and super-fast HTTP parser written in C for the Node.js project
It's only 2 C files, without any external dependencies.
Solution 2
The problem in the code you've posted is pretty simple: strtok
works by modifying the string you pass to it. Modifying a string literal gives undefined behavior. A truly minuscule change to your code lets it work (I've also headed the appropriate headers, and moved the executable part into a function:
#include <string.h>
#include <stdio.h>
char response[] = "HTTP/1.1 200 OK\nServer: Apache-Coyote/1.1\nPragma: no-cache";
int main() {
char *token = NULL;
token = strtok(response, "\n");
while (token) {
printf("Current token: %s.\n", token);
token = strtok(NULL, "\n");
}
return 0;
}
In real use, you'll be reading the HTTP response into a buffer anyway; the problem you encountered only arises in a trivial test case like you generated. At the same time, it does point to the fact that strtok
is a pretty poorly designed function, and you'd almost certainly be better off with something else.
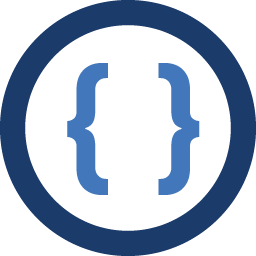
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I'm writing a little project which interacts with a set of servers using HTTP 1.1 GET and POST. The server gives me the response after some header lines, so I though on using
strtok()
function using\n
as the delimiter but there is a crash whenever I try to do so.Is there any simple way to parse a HTTP response in C? I would like not to use 3rd party libraries for this but if it was really necesary I won't have any problem.
Thank you very much for everything.
EDIT: Here is some example code, just trying to print the lines:
char *response = "HTTP/1.1 200 OK\nServer: Apache-Coyote/1.1\nPragma: no-cache" char *token = NULL; token = strtok(response, "\n"); while (token) { printf("Current token: %s.\n", token); token = strtok(NULL, "\n"); }
-
R Samuel Klatchko about 14 yearsNot sure why you think this issue reflects poorl on strtok. The real problem is the fact that a string literal is considered a
char *
instead of aconst char *
and the issue can affect any code that modifies the string input. -
Jerry Coffin about 14 yearsIt reflects poorly on
strtok
, because it unnecessarily restricts its range of input to data that's not only modifiable, but that you don't mind basically destroying in the process of using it. -
Elazar Leibovich about 9 yearsIt's written in callback-style to support
poll
-like loops. Maybe the author is interested with blocking parser, likegolang
's ReadRequest -
Bharat about 7 yearsIs there an example client written using this parser in C? Examples which come with the repository don't seem sufficient for a novice like me.