How to pass parameters to an eval based function inJavascript
11,261
Solution 1
Use Function constructor
var body = "console.log(arguments)"
var func = new Function( body );
func.call( null, 1, 2 ); //invoke the function using arguments
with named parameters
var body = "function( a, b ){ console.log(a, b) }"
var wrap = s => "{ return " + body + " };" //return the block having function expression
var func = new Function( wrap(body) );
func.call( null ).call( null, 1, 2 ); //invoke the function using arguments
Solution 2
Eval evaluates whatever you give it to and returns even a function.
var x = eval('(y)=>y+1');
x(3) // return 4
So you can use it like this:
var lim = 3;
var body = 'return lim+1;';
JSON.stringify(eval('(lim) => {' + body + '}')(lim)); //returns "4"
Another way using Function:
var lim = 3;
JSON.stringify((new Function('lim', this.selectedFunction.body))(lim));
Solution 3
You can use the Function object.
var param1 = 666;
var param2 = 'ABC';
var dynamicJS = 'return `Param1 = ${param1}, Param2 = ${param2}`';
var func = new Function('param1', 'param2', dynamicJS);
var result = func(param1, param2);
console.log(result);
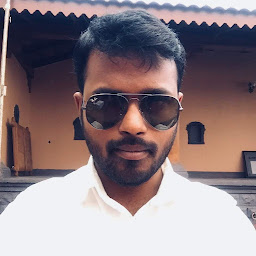
Comments
-
Saket Nalegaonkar almost 2 years
I am storing function body in string with function name.
function fnRandom(lim){ var data=[]; for(var i=0;i<lim;i++) { data=data.concat(Math.floor((Math.random() * 100) + 1)); } return data; }
After selecting the functionName from a drop down I use eval to execute function body.
JSON.stringify(eval(this.selectedFunction.body));
I want to pass 'lim' to this execution or can I use functionName as initiating point for execution somehow?
-
Barmar about 6 yearsYou didn't specify any arguments in the
new Function()
call. -
Barmar about 6 yearsThis only works if the function uses
arguments
instead of named parameters. -
Barmar about 6 yearshe only has the function body, not the whole function.
-
gurvinder372 about 6 years@Barmar yes, I have added a version with named parameters as well.
-
Barmar about 6 yearsYour function body is the whole function, not just the body.
-
Barmar about 6 yearsWhat if the parameter isn't named
lim
? -
Gerry about 6 yearsHe has to know how his parameters have to be named in his body-strings. Otherwise @gurvinder372 's answer will suit better.
-
Barmar about 6 yearsYes, I think he has some fundamental design problems because of that. A solution like this doesn't work with arbitrary functions. He really needs to store more information in
this.selectedFunction
than just the body. -
gurvinder372 about 6 yearsIf function is only body in OP's case, then named parameters cannot be used since we don't know the names of parameters used in the body
-
Gerry about 6 yearsI fully agree with you
-
Barmar about 6 yearsI know, I wrote that in a comment above. I think he has a design problem that makes this impossible to solve in a general way.