How to pass Windows Authentication credential from client to Web API service
Solution 1
If you use impersonation on your web site and the API is running on the same server it should work.
http://msdn.microsoft.com/en-us/library/aa292118(v=vs.71).aspx
However, if you would move the API to a different server from the site this will stop working. A two-server setup requires Kerberos delegation.
Solution 2
Settings for web API
- Enable
Windows Authentication
Settings for web application
- Enable
Windows Authentication
- Add
<identity impersonate="true" />
in<system.web>
of web.config -
Add the following in the web.config:
<system.webServer> <validation validateIntegratedModeConfiguration="false" /> </system.webServer>
Enable
Windows Authentication
andASP.NET Impersonation
within IIS
You can use the following code to POST data to web API (and GET as well obviously)
using (var client = new WebClient { UseDefaultCredentials = true })
{
client.Headers.Add(HttpRequestHeader.ContentType, "application/xml; charset=utf-8");
byte[] responseArray = client.UploadData("URL of web API", "POST", Encoding.UTF8.GetBytes(XMLText));
string response = Encoding.ASCII.GetString(responseArray);
}
NOTE: If you're still getting 401 errors you may need to use an IP address instead of a regular domain name for your URL (e.g.: 155.100.100.10 instead of mycompany.com)
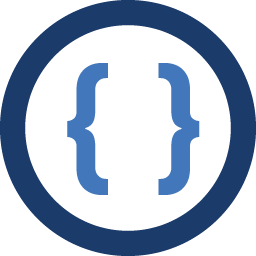
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
Inside my corporate environment, I have IIS7.5 hosting both a Web API service and a separate website which makes calls into that service via the RestSharp library. Both are currently configured with Windows Authentication.
If I navigate to either one with a browser, I'm prompted to enter my windows credential, and everything works great... I get web pages that I want and the REST service spits out my data. The part I'm struggling to figure out is how to use a single credential to authentication both. I can't figure out how to either pass the Website's credential to the service (I tried impersonating but it didn't work), or to manually prompt the user for username/password and then authenticate them with "Windows".
Help a noob out?
-
Admin over 9 yearsThanks, @Anders. The site and API are running on different sites. I'll take a look at kerberos.
-
Anders Bornholm over 9 yearsI'd like to warn you that Kerberos delegation is non-trivial. I've yet to find a single IT department that knows how to do it or are willing to try. A workaround is to use a service account that has access to everything that the site can use when calling the API server-to-server. It's not the prettiest solution and it requires that you check in code that the user logged in to the web site doesn't get more information from the API than he or she is supposed to. But at least it's a working option that doesn't require a lot of intrusive changes made to the IT environment.