How to place Admob Native Advanced Ads in recycler view android?
Solution 1
first you create the Ads continer item_ads.xml folder
<com.google.android.ads.nativetemplates.TemplateView
android:id="@+id/my_template"
<!-- this attribute determines which template is used. The other option is
@layout/gnt_medium_template_view -->
app:gnt_template_type="@layout/gnt_small_template_view"
android:layout_width="match_parent"
android:layout_height="match_parent" />
secend in The adapter you must change the extends to
extends RecyclerView.Adapter<RecyclerView.ViewHolder>
now you need to override 4 methods
public RecyclerView.ViewHolder onCreateViewHolder
public void onBindViewHolder
public int getItemCount()
public int getItemViewType
in the getItemViwType methode we define two possibilities
@Override
public int getItemViewType(int position) {
if (AD_LOGIC_CONDITION)) {
return AD_TYPE;
}else{
return CONTENT_TYPE; ///do not forget to initialize bouth of AD_TYPE and CONTENT_TYPE
}
then we create two view holder one for your content and secend for our Ad I will assume that you know how to create your view holder so i will just explain the AD View holder
class adViewHolder extends RecyclerView.ViewHolder {
TemplateView Adtemplate;
public adViewHolder(@NonNull View itemView) {
super(itemView);
Adtemplate = itemView.findViewById(R.id.my_template);
}
now we return to the onCreateViewHolder method
public RecyclerView.ViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
if (viewType == AD_TYPE) {
adViewHolder madViewHolder = new adViewHolder(LayoutInflater.from(parent.getContext()).inflate(R.layout.item_ads, null, false));
return madViewHolder;
} else{
YourViewHolder mYourViewHolder = new YourViewHolder(LayoutInflater.from(parent.getContext()).inflate(R.layout.recycler_item, null, false));
return mYourViewHolder;
}
now we go to the onBindViewHolder
@Override
public void onBindViewHolder(@NonNull final RecyclerView.ViewHolder holder, int position) {
if (getItemViewType(position) == TYPE_CONTENT) {
///your data
// AN EXAMPLE
((YourViewHolder) holder).textview.setText(data.getmtext());
((YourViewHolder) holder).Img.setImageResource(data.getmImg());
((YourViewHolder) holder).title.setText(data.getmName());
} else if (getItemViewType(position) == TYPE_AD){
final AdLoader adLoader = new AdLoader.Builder(context, "ca-app-pub-3940256099942544/2247696110")
.forUnifiedNativeAd(new UnifiedNativeAd.OnUnifiedNativeAdLoadedListener() {
@Override
public void onUnifiedNativeAdLoaded(UnifiedNativeAd unifiedNativeAd) {
// Show the ad.
NativeTemplateStyle styles = new
NativeTemplateStyle.Builder().build();
TemplateView template = ((adViewHolder) holder).Adtemplate;
template.setStyles(styles);
template.setNativeAd(unifiedNativeAd);
}
})
.withAdListener(new AdListener() {
@Override
public void onAdFailedToLoad(int errorCode) {
// Handle the failure by logging, altering the UI, and so on.
}
})
.withNativeAdOptions(new NativeAdOptions.Builder()
// Methods in the NativeAdOptions.Builder class can be
// used here to specify individual options settings.
.build())
.build();
adLoader.loadAd(new AdRequest.Builder().build());
}
Solution 2
MainActivity.java
RecyclerView rvContacts = (RecyclerView) findViewById(R.id.rvContacts);
// Initialize contacts
contacts = Contact.createContactsList(20);
// Create adapter passing in the sample user data
ContactsAdapter adapter = new ContactsAdapter(contacts);
//Build the native adapter from the current adapter
AdmobNativeAdAdapter admobNativeAdAdapter=AdmobNativeAdAdapter.Builder.with(
"ca-app-pub-3940256099942544/2247696110",//admob native ad id
adapter,//current adapter
"small"//Set the size "small", "medium" or "custom"
).adItemInterval(5)//Repeat interval
.build();
// Attach the new adapter to the recyclerview to populate items
rvContacts.setAdapter(admobNativeAdAdapter);
// Set layout manager to position the items
rvContacts.setLayoutManager(new LinearLayoutManager(this));
}
MORE READ RecyclerView-Native-ads-Example-Github
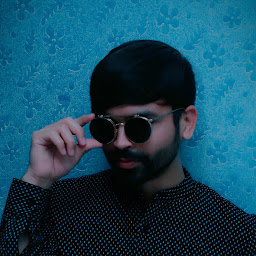
Rohit Kumar Sehrawat
Updated on June 17, 2022Comments
-
Rohit Kumar Sehrawat almost 2 years
I want place the admob native advanced ads in every 3 position of my recycler view in android app.
I would like to template provided by Admob.
https://github.com/googleads/googleads-mobile-android-native-templates
Here is xml code implementation of native ads
<com.google.android.ads.nativetemplates.TemplateView android:id="@+id/my_template" <!-- this attribute determines which template is used. The other option is @layout/gnt_medium_template_view --> app:gnt_template_type="@layout/gnt_small_template_view" android:layout_width="match_parent" android:layout_height="match_parent" />
Here is Java code implementation of Admob
MobileAds.initialize(this, "[_app-id_]"); AdLoader adLoader = new AdLoader.Builder(this, "[_ad-unit-id_]") .forUnifiedNativeAd(new UnifiedNativeAd.OnUnifiedNativeAdLoadedListener() { @Override public void onUnifiedNativeAdLoaded(UnifiedNativeAd unifiedNativeAd) { NativeTemplateStyle styles = new NativeTemplateStyle.Builder().withMainBackgroundColor(background).build(); TemplateView template = findViewById(R.id.my_template); template.setStyles(styles); template.setNativeAd(unifiedNativeAd); } }) .build(); adLoader.loadAd(new AdRequest.Builder().build()); }
RecyclerView Adapter Class:
public class ArticleAdapter extends RecyclerView.Adapter<ArticleAdapter.MyViewHolder>{ private Context mContext; private List<ArticleJson> articleList; String titleoflist; public class MyViewHolder extends RecyclerView.ViewHolder { TextView txtTitle,txtDesc,txtStatus,txtColor,txtAuthor; LinearLayout linearLayout; private ArticleJson m_articleJson; public MyViewHolder(View view) { super(view); txtTitle = view.findViewById(R.id.texViewArticleTitle) linearLayout = view.findViewById(R.id.article_linearlayout); } public void bindView(final ArticleJson articleJson){ m_articleJson = articleJson; txtTitle.setText(articleJson.getmTitle()); txtAuthor.setText(titleoflist); } } public ArticleAdapter(Context mContext, List<ArticleJson> articleList,String titleoflist) { this.mContext = mContext; this.articleList = articleList; this.titleoflist = titleoflist; } @NonNull @Override public ArticleAdapter.MyViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) { View itemView = LayoutInflater.from(parent.getContext()) .inflate(R.layout.list_item_article, parent, false); return new ArticleAdapter.MyViewHolder(itemView); } @Override public void onBindViewHolder(@NonNull ArticleAdapter.MyViewHolder holder, int position) { final ArticleJson articleJson = articleList.get(position); holder.bindView(articleJson); holder.linearLayout.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { //Toast } }); } @Override public int getItemCount() { return articleList.size(); } }
-
GGK stands for Ukraine almost 4 yearsThere is no question, so no one can help
-
Rohit Kumar Sehrawat almost 4 yearsi have edited my question
-
-
Rohit Kumar Sehrawat almost 4 yearsThanks I understand the concept but i don't understand the code. because i didn't find native ads template implementation in it. Also getItemViewType look confusing!!!
-
Rohit Kumar Sehrawat over 3 yearsWould you mind to help me this stackoverflow.com/q/63335533/4499768
-
Fish over 3 yearsIt works, but its not a nice approach. Ads does not load until the user scroll. You have scroll back up in order to see an ad. Therefore it is recommended to load ad with
List<UnifiedNativeAd>
. -
Kishan Solanki over 2 yearsThis works but have you tried notifyItemRemoved on any of the adapter? Its not working.