How to play animation on div with javascript
13,831
Solution 1
Try to add 'animation' css property from js:
document.getElementById('test').style.animation = 'fading 2s infinite'
Solution 2
Add the animation to a class in CSS.
.fade {
animation: fading 1s forwards; // "fading" is the keyframe animation you created
}
[forwards][1]
makes it so the element remains in the final state of the animation.
Then in Javascript when you want to animate your div, add the class to the element.
var el = document.getElementById('test'); // get a reference to the targeted element
el.classList.add('fade'); // add the class name to that element
document.getElementById('fader').addEventListener('click', function() {
var el = document.getElementById('test');
el.classList.add('fade');
});
#test {
background-color: blue;
width: 100px;
height: 100px;
}
.fade {
animation: fading 1s forwards;
}
/* Here is the animation (keyframes) */
@keyframes fading {
0% {
opacity: 1;
}
100% {
opacity: 0;
}
}
<div id="test"></div>
<button type="button" id="fader">fade out</button>
Solution 3
You have to add the animation keyframe fading
to the div
.
Have a look at this
#test {
background-color: blue;
width: 100px;
height: 100px;
position: relative;
-webkit-animation: fading 5s infinite;
animation: fading 5s infinite;
}
/* Here is the animation (keyframes) */
@keyframes fading {
0% { opacity: 1; }
100% { opacity: 0; }
}
<html>
<head>
<link rel="stylesheet" type="text/css" href="mystyles.css">
</head>
<body>
<div id="test"></div>
</body>
</html>
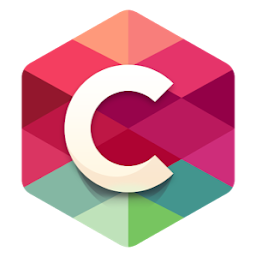
Author by
Clint Anumudu
Updated on June 14, 2022Comments
-
Clint Anumudu almost 2 years
Here is my script so far:
Html:
<head> <link rel="stylesheet" type="text/css" href="mystyles.css"> </head> <body> <div id="test"></div> </body> </html>
Css:
#test { background-color: blue; width: 100px; height: 100px; } /* Here is the animation (keyframes) */ @keyframes fading { 0% { opacity: 1; } 100% { opacity: 0; } }
But how do i get the css animation (keyframes) to play on the div #test using some javascript?
-
Nope over 6 yearsAssign your
@keyframes fading
to a class and use JavaScript to add/remove the class as needed - css-tricks.com/snippets/css/keyframe-animation-syntax -
Core972 over 6 yearsHere a tuto link
-
Nope over 6 yearsWhy would you link w3fools if there is a billion professional sites and original documentation....
-
Saurabh Mistry over 6 yearsread more about animation here : w3schools.com/howto/howto_js_animate.asp
-
Randall over 6 yearsIf you get the right answer apply it clicking in the check button.
-