How to Prevent "AttributeError: 'function' object has no attribute ''"
You are receiving an error because alphabet is a variable defined within a function. It is therefore a local variable which cannot be accessed outside of that function.
I am very confused as to why there is a function there in the first place. I would replace everything under the alphabet function with just the variable containing the characters in order.
There are actually quite a few things wrong with this code - the code in the for loop is flawed as well. Here is my solution, with the added benefit of printing the encoded message as one string and not as a load of characters, as well as dealing with non-alphanumeric characters.
elif option1 == 2:
alphabet = 'abcdefghijklmnopqrstuvwxyz'
message = input('What message would you like to encrypt?')
encoded = []
for character in message:
if character.isalpha():
encoded.append(alphabet[25-alphabet.find(character)])
else:
encoded.append(character)
print(''.join(encoded))
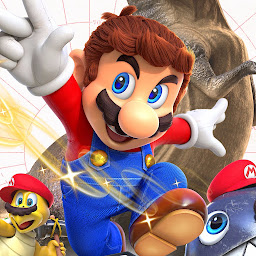
Mariofan717
Updated on June 04, 2022Comments
-
Mariofan717 almost 2 years
I have been working on a program that is meant to encrypt messages inputted by the user. There are two encryption options, and the second one is meant to make each character in the message what it would be if the alphabet was reversed. When I input a message, I am given the error "AttributeError: 'function' object has no attribute 'find'".
elif option1 == 2: def alphabet(): alphabet = 'abcdefghijklmnopqrstuvwxyz' 'abcdefghijklmnopqrstuvwxyz'.reverse() == 'zyxwvutsrqponmlkjihgfedcba' 'abcdefghijklmnopqrstuvwxyz' [::-1] == 'zyxwvutsrqponmlkjihgfedcba' message = raw_input('What message would you like to encrypt?') message = raw_input('What message would you like to encrypt?') for character in message: position = alphabet.find(character) newPosition = (position) % 26 newCharacter = alphabet[newPosition] print(newCharacter)enter code here
I structured it similarly to the code for the first option which runs without any issues, and I am aware that this error may have originated from the def statement which I am not entirely sure how to properly structure.
if option1 == 1: add = 13 message = raw_input('What message would you like to encrypt?') for character in message: if character in alphabet: position = alphabet.find(character) newPosition = (position + add) % 26 newCharacter = alphabet[newPosition] print(newCharacter)
I understand that questions such as this are frequently asked on this site, but the answers to those have not helped me, as I have very little programming experience.
-
Nir Alfasi over 6 years
def alphabet():
<-- it means thatalphabet
is a function, what did you mean by writing:alphabet.find(...)
?
-
-
Mariofan717 over 6 yearsI trust that the replacement code you have written will function after this is resolved, but after inputting the message I am given "NameError: name '(message)' is not defined".
-
Daniel over 6 yearsYou can also use
str.ascii_lowercase
!