How to print a signed integer as hexadecimal number in two's complement with python?
14,773
Solution 1
Here's a way (for 16 bit numbers):
>>> x=-123
>>> hex(((abs(x) ^ 0xffff) + 1) & 0xffff)
'0xff85'
(Might not be the most elegant way, though)
Solution 2
>>> x = -123
>>> bits = 16
>>> hex((1 << bits) + x)
'0xff85'
>>> bits = 32
>>> hex((1 << bits) + x)
'0xffffff85'
Solution 3
Using the bitstring module:
>>> bitstring.BitArray('int:32=-312367').hex
'0xfffb3bd1'
Solution 4
Simple
>>> hex((-4) & 0xFF)
'0xfc'
Solution 5
To treat an integer as a binary value, you bitwise-and it with a mask of the desired bit-length.
For example, for a 4-byte value (32-bit) we mask with 0xffffffff
:
>>> format(-1 & 0xffffffff, "08X")
'FFFFFFFF'
>>> format(1 & 0xffffffff, "08X")
'00000001'
>>> format(123 & 0xffffffff, "08X")
'0000007B'
>>> format(-312367 & 0xffffffff, "08X")
'FFFB3BD1'
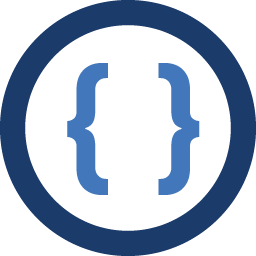
Author by
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I have a negative integer (4 bytes) of which I would like to have the hexadecimal form of its two's complement representation.
>>> i = int("-312367") >>> "{0}".format(i) '-312367' >>> "{0:x}".format(i) '-4c42f'
But I would like to see "FF..."
-
dcoles over 8 yearsThis doesn't work for positive numbers. If x=1 you get
0xffff
(-1 in 16-bit two's complement), instead of0x0001
. -
dcoles over 8 yearsFor 123, isn't the 16-bit 2's complement representation 0x007b? 0xff85 would be for -123.
-
John La Rooy over 8 years@dcoles, no the sum of 123 and it's 2's complement should be 0 (the carry/overflow bit is discarded) It doesn't matter whether you want to treat
0xff85
as signed or unsigned. -
dcoles over 8 yearsI agree that the 2's complement (the operation on binary numbers) of 0x007b is 0xff85, but I believe the question was about the 2's complement signed number representation.
-
John La Rooy over 8 years@dcoles, ah. I think I get what you are saying. It's proably clearer if I make
x = -123
-
dcoles over 8 yearsThe example given was a negative integer, but the question was "How to print a signed integer... in two's complement [representation]". It could be confusing if this answer only works for negative signed integers.