How to print Firestore timestamp as formatted date and time
Solution 1
When we push the DateTime object to Firestore, it internally converts it to it's own timestamp object and stores it.
Method to convert it back to Datetime after fetching timestamp from Firestore:
Firestore's timestamp contains a method called toDate() which can be converted to String and then that String can be passed to DateTime's parse method to convert back to DateTime
DateTime.parse(timestamp.toDate().toString())
Solution 2
Here is way!
Firestore will return TimeStamp like Timestamp(seconds=1560523991, nanoseconds=286000000).
This can be parsed as
Timestamp t = document['timeFieldName'];
DateTime d = t.toDate();
print(d.toString()); //2019-12-28 18:48:48.364
Solution 3
You can directly convert the Firestore timestamp object to DateTime like this:
DateTime myDateTime = (snapshot.data.documents[index].data['timestamp']).toDate();
This will return your Firestore timestamp in the dart's DateTime format. In order to convert your DateTime
object you can use DateFormat
class from intl package.
You can use your obtained DateTime object to get the format of your choice like this:
DateFormat.yMMMd().add_jm().format(myDateTime);
This code produces an output like this:
Apr 21, 2020 5:33 PM
Solution 4
timestamp parameter is the time in seconds
String formatTimestamp(int timestamp) {
var format = new DateFormat('d MMM, hh:mm a');
var date = new DateTime.fromMillisecondsSinceEpoch(timestamp * 1000);
return format.format(date);
}
Please check this answer for intl date formats
Hope it helps !
Solution 5
I found Ashutosh's suggestion gives more user friendly output. A function like this is recommended in a helper class with a static method.
static convertTimeStamp(Timestamp timestamp) {
assert(timestamp != null);
String convertedDate;
convertedDate = DateFormat.yMMMd().add_jm().format(timestamp.toDate());
return convertedDate;
}
Intl package is require for DateFormat
.
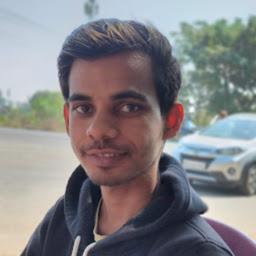
aryan singh
Updated on August 11, 2021Comments
-
aryan singh over 2 years
My timestamp returns
Timestamp(seconds=1560523991, nanoseconds=286000000)
in a Flutter Firestore snapshot.I want to print it as properly formatted date and time.
I'm using
DateTime.now()
to store current DateTime in Firestore while creating new records and retrieving it using Firestore snapshot but I am not able to convert to into formatted date time. I'm using libintl.dart
for formatting.Code for saving data
d={'amount':amount, 'desc':desc, 'user_id':user_id, 'flie_ref':url.toString(), 'date':'${user_id}${DateTime.now().day}-${DateTime.now().month}-${DateTime.now().year}', 'timestamp':DateTime.now() return Firestore.instance.collection('/data').add(d).then((v){return true; }).catchError((onError)=>print(onError)); }); Accessing with FutureBuilder( future: Firestore.instance .collection('data') .where('user_id', isEqualTo:_user_id) .getDocuments(), builder: (BuildContext context, AsyncSnapshot<QuerySnapshot> snapshot) { if (!snapshot.hasData) return Container( child: Center(child: CircularProgressIndicator())); return ListView.builder( itemCount: snapshot.data.documents.length, itemBuilder: (BuildContext context, int index) { return Column( children: <Widget>[ Text(DateFormat.yMMMd().add_jm().format(DateTime.parse(snapshot.data.documents[index].data['timestamp'].toString())]); ....
Error thrown is
Invalid date format.
I'm expecting output is:
'Jan 17, 2019, 2:19 PM'