How to print the string representation of an "enum" in Go?
11,276
You can't directly within the language, but there's a tool for generating the supporting code: golang.org/x/tools/cmd/stringer
From the example in the stringer
docs
type Pill int
const (
Placebo Pill = iota
Aspirin
Ibuprofen
Paracetamol
Acetaminophen = Paracetamol
)
Would produce code like
const _Pill_name = "PlaceboAspirinIbuprofenParacetamol"
var _Pill_index = [...]uint8{0, 7, 14, 23, 34}
func (i Pill) String() string {
if i < 0 || i+1 >= Pill(len(_Pill_index)) {
return fmt.Sprintf("Pill(%d)", i)
}
return _Pill_name[_Pill_index[i]:_Pill_index[i+1]]
}
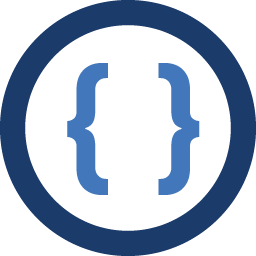
Author by
Admin
Updated on June 15, 2022Comments
-
Admin about 2 years
I've looked at the various official sources for how to do this but I can't find it. Imagine you have the following enum (I know golang doesn't have enums in the classic sense):
package main import "fmt" type LogLevel int const ( Off LogLevel = iota Debug ) var level LogLevel = Debug func main() { fmt.Printf("Log Level: %s", level) }
The closest I can get with the above
%s
, which gives me:Log Level: %!s(main.LogLevel=1)
I would like to have:
Log Level: Debug
Can anyone help me?