How to println a string and variable within the same parameters
Solution 1
You either need to use + to concatenate, or use String.format to format a string or similar.
The easiest is to do concatenation:
System.out.println("randomtext" + var);
Some people often use System.out.printf
which works the same way as String.format
if you have a big messy string you want to concatenate:
System.out.printf("This is %s lot of information about %d and %s", var, integer, stringVar);
When using String.format the most important formatters you need to know is %s
for Strings, %f
for floating-point numbers, %d
for integers.
More information about how to use String.format can be found in the documentation about Formatter.
Solution 2
You can concatenate the two strings together; the overloaded println
methods take at most one parameter.
System.out.println("randomtext" + var);
Solution 3
System.out.println(String.format("randomtext %s", var));
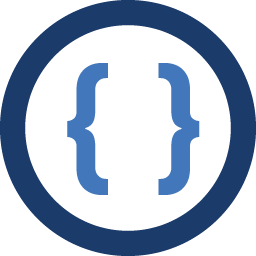
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I am writing some code right now and I haven't done this in a while, how would I use the println function to write both a string and a variable?
I tried the following:
System.out.println("randomtext"var);
and
System.out.println("randomtext",var);
Neither of those seemed to work, what am I doing wrong? How do I do this?