How to programmatically switch tabs using buttonclick in Android
Solution 1
Here's a code example that you can put inside your onClick(). It's as Mark and Kevin described.
TabActivity tabs = (TabActivity) getParent();
tabs.getTabHost().setCurrentTab(2);
I've used this code tidbit numerous times. Hope this clarifies.
Solution 2
There is no widget with @android:id/tabhost
in the current activity. Hence, findViewById()
returns null, and your call to setCurrentTab()
fails.
Now, my guess is that is because you are putting activities in your tabs. Had you put Views
in your tabs, your code would work. Your code would also be faster, take up less heap space, and be at reduced risk of running out of stack space.
If you wish to stick with your current implementation, try calling getParent().findViewById()
instead of just findViewById()
.
Solution 3
2017 answer
The other answers here appear to be outdated. The Creating Swipe Views with Tabs documentation recommends using TabLayout
with a ViewPager
.
Here is one partial implementation of the code to do this.
public class MainActivity extends AppCompatActivity {
private ViewPager mViewPager;
private Button mButton;
@Override
public void onCreate(Bundle savedInstanceState) {
// ...
mViewPager = (ViewPager) findViewById(R.id.fieldspager);
// ...
mButton.setOnClickListener(myButtonClickHandler);
}
View.OnClickListener myButtonClickHandler = new View.OnClickListener() {
@Override
public void onClick(View view) {
mViewPager.setCurrentItem(2, true);
}
};
}
From the code above, setting the tab programmatically is done like this:
mViewPager.setCurrentItem(2, true); // set it to the third tab
Here is another implementation with more details of how the view pager and tab layout are set up.
Solution 4
This works for me
getActionBar().setSelectedNavigationItem(0);
Update: plugging my code into the question's code to give it more context...
ImageButton next = (ImageButton) findViewById(R.id.ButtonAsk);
next.setOnClickListener(new View.OnClickListener()
{
public void onClick(View view)
{
getActionBar().setSelectedNavigationItem(2);
}
});
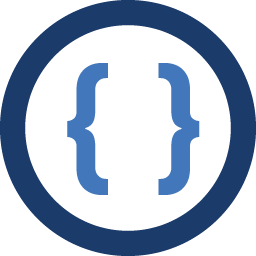
Admin
Updated on February 07, 2020Comments
-
Admin over 4 years
I have been struggling with this for a few days now. I'm trying to switch tabs programmatically upon a button click. My program works flawlessly if I just use the tabs to change activities, but wiring an onClick method with setCurrentTab results in an error. This is the method that will not work. It's a pretty basic and straightforward function but I haven't seen much documentation or examples of people attempting to wire a buttonclick with switching tabs. Thanks.
ImageButton next = (ImageButton) findViewById(R.id.ButtonAsk); next.setOnClickListener(new View.OnClickListener() { public void onClick(View view) { TabHost tabHost = (TabHost) findViewById(android.R.id.tabhost); tabHost.setCurrentTab(2); } });
See the edit history for the error log.