How to properly use minify maven plugin to minify js and css in Angularjs app?
Solution 1
You are on the right track.
Note that when you minify a JavaScript code of a controller, all of its function arguments would be minified as well, and the dependency injector would not be able to identify services correctly.
It's possible to overcome this problem by annotating the function with the names of the dependencies, provided as strings, which will not get minified. There are two ways of doing it:
(1.) Create a $inject
property on the controller function which holds an array of strings. For example:
function MyController($scope, $filter, $location, $interval, ngTableParams, $modal, $transition, myService, $timeout) {...}
MyController.$inject = ['$scope', '$filter', '$location', '$interval', 'ngTableParams', '$modal', '$transition', 'myService', '$timeout'];
(2.) Use an inline annotation where, instead of just providing the function, you provide an array. In your case it would look like:
angular.module('myApp').controller('MyController', ['$scope', '$filter', '$location', '$interval', 'ngTableParams', '$modal', '$transition', 'myService', '$timeout', function($scope, $filter, $location, $interval, ngTableParams, $modal, $transition, myService, $timeout) {
...
}]);
For more info please check out "A Note on Minification" section of this tutorial.
Solution 2
Also be careful with reserved words in mvn, like 'then' and 'catch'.
$http.get('some.json').then(convertResponse).catch(throwError);
Might be rewritten as:
$http.get('some.json')['then'](convertResponse)['catch'](throwError);
Hopefully somebody can provide a better solution, this looks really nasty.
More on Missing name after . operator YUI Compressor for socket.io js files
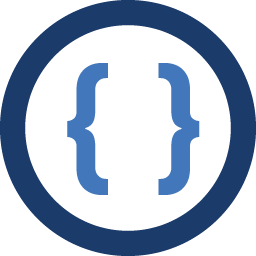
Admin
Updated on July 26, 2022Comments
-
Admin almost 2 years
I am trying to minify javascripts and css files in my angularjs app using samaxes minify maven plugin. I am able to get all js & css minified and build a war file with maven, but while trying to open app url I get
Error: [$injector:unpr] Unknown provider: aProvider <- a
and my app does not work.Below I am providing my pom plugin configuration
<plugin> <groupId>com.samaxes.maven</groupId> <artifactId>minify-maven-plugin</artifactId> <version>1.7.4</version> <executions> <execution> <id>min-js</id> <phase>prepare-package</phase> <goals> <goal>minify</goal> </goals> </execution> </executions> <configuration> <charset>UTF-8</charset> <skipMerge>true</skipMerge> <cssSourceDir>myapp/styles</cssSourceDir> <jsSourceDir>myapp/javascript</jsSourceDir> <jsEngine>CLOSURE</jsEngine> <closureLanguage>ECMASCRIPT5</closureLanguage> <closureAngularPass>true</closureAngularPass> <nosuffix>true</nosuffix> <webappTargetDir>${project.build.directory}/minify</webappTargetDir> <cssSourceIncludes> <cssSourceInclude>**/*.css</cssSourceInclude> </cssSourceIncludes> <cssSourceExcludes> <cssSourceExclude>**/*.min.css</cssSourceExclude> </cssSourceExcludes> <jsSourceIncludes> <jsSourceInclude>**/*.js</jsSourceInclude> </jsSourceIncludes> <jsSourceExcludes> <jsSourceExclude>**/*.min.js</jsSourceExclude> </jsSourceExcludes> </configuration> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-war-plugin</artifactId> <version>2.4</version> <configuration> <webResources> <resource> <directory>${project.build.directory}/minify</directory> </resource> </webResources> </configuration> </plugin>
Directory structure
My controller structure
'use strict'; angular.module('myApp').controller('MyController', function($scope, $filter, $location, $interval, ngTableParams, $modal, $transition, myService, $timeout) { ... });
Chrome console error
Does samaxes minify maven plugin support minifying angularjs apps or do I need to use any other alternatives?
Please help me in minifying js and css in my angularjs app.