How to read comma separated values from a text file, then output result to a text file?
Solution 1
You have the right idea going, let's start by opening some files:
with open("text.txt", "r") as filestream:
with open("answers.txt", "w") as filestreamtwo:
Here, we have opened two filestreams - "text.txt" and "answers.txt".
Since we used with
, these filestreams will automatically close after the code that is indented beneath them finishes running.
Now, let's run through the file "text.txt" line by line:
for line in filestream:
This will run a for loop and end at the end of the file.
Next, we need to change the input text into something we can work with, such as an array:
currentline = line.split(",")
Now, currentline
contains all the integers listed in the first line of "text.txt".
Let's sum up these integers:
total = str(int(currentline[0]) + int(currentline[1]) + int(currentline [2])) + "\n"
We had to wrap each element in currentline
with the int
function around. Otherwise, instead of adding the integers, we would be concatenating strings!
Afterwards, we add the carriage return, "\n"
in order to make "answers.txt" clearer to understand.
filestreamtwo.write(total)
Now, we are writing to the file "answers.txt"... That's it! You're done!
Here's the code again:
with open("test.txt", "r") as filestream:
with open("answers.txt", "w") as filestreamtwo:
for line in filestream:
currentline = line.split(",")
total = str(int(currentline[0]) + int(currentline[1]) + int(currentline [2])) + "\n"
filestreamtwo.write(total)
Solution 2
You can do this in fewer lines, but I hope you find this solution readable and easy to understand:
out = file('answers.txt', 'w')
for line in file('file.txt', 'r'):
s = 0
for num in line.strip().split(','):
s += int(num)
out.write("%d\n" % s)
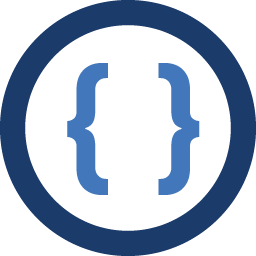
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I need to create a program that will add numbers read from a text file separated by commas. i.e.
in
file.txt
:1,2,3 4,5,6 7,8,9
So far I have the simple code:
x = 1 y = 2 z = 3 sum = x + y + z print(sum)
I'm not sure how I would assign each number in the text file to
x
,y
andz
.What I would like is that it will iterate through each line in the text file, this would be with a simple loop.
However I also do not know how I would then output the results to another text file. i.e.
answers.txt
:6 15 24
-
l4mpi over 10 yearsThis is neither code nor answer golf. Downvoted as I don't consider your answer helpful, especially not for a new programmer.
-
EducateMe over 10 yearsHi l4mpi, Thanks for the response, the OP stated they wanted the file output in a .txt format so I avoided using csv. I forgot about "for line in file", I will amend my answer with that now. I'm not familiar with "with", could you please respond with an example of how to open a filestream using "with", as the responses I have found online are very complex for me. I have only used python, and as you've said, not very well. I originally came to stackoverflow to learn java, but I guess I might be better off spending more time in /python!
-
l4mpi over 10 yearsYou seem to be confused - a csv file is just a normal text file, and you can save it with any ending you want including
.txt
. As for with statements in the context of files, you dowith open("myfile.txt") as f
and can then use the file object in the block of the with statement; it will be automatically closed after the block. See the other answers for examples of that. -
EducateMe over 10 yearsIs this the best implementation? pastebin.com/6PyxetVz - Should I use map for the ints?
-
l4mpi over 10 years"The best" is somewhat subjective, but I'd say it's way better than your current code and definitely a good solution to OPs problem. Edit that in and you'll get an upvote :)
-
Joran Beasley over 10 yearsMeh ask for code with little to no effort and I'll play golf... It looks to me like he spent more time writing his question than he did on his code ...
-
deadcode about 6 yearsMaybe @EducateMe didn't write the best code but his patience and explanations seems great for beginners wanting to learn and we are in need of patient educators like this.