How to read from a USB RFID Reader with C#
It's most likely in the package LibUsbDotNet
.
If you are using Visual Studio, open up Tools > NuGet Package Manager > Manage NuGet Packages for Solution..., go to Browse and paste it into the search bar. Add it to your project and now it should be good to go.
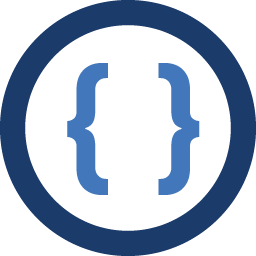
Admin
Updated on July 14, 2022Comments
-
Admin almost 2 years
I just bought an USB RFID Reader and now I want to read, write and check if a card is on the Reader at the moment or not. So I searched here and found the Code below. The description of it is pretty much all what I Need:
Opens a USB device by vendor and product id.
Opens a UsbEndpointReader class for reading.
Reads and displays usb device output from Ep01 until no data is received for 5 seconds.
But I dont understand where "USBDevice", "USBDeviceFinder" etc. are coming from? It's the only Code the user posted. I copied it into my Visual Studio and as expected it says for example "The Name 'USBDevice' does not exist in the current context"
Can someone help how to fix this?
Btw. this is the original question and the answers: Link to the Original Post
using System; using System.Text; using LibUsbDotNet; using LibUsbDotNet.Main; namespace Examples { internal class ReadPolling { public static UsbDevice MyUsbDevice; #region SET YOUR USB Vendor and Product ID! public static UsbDeviceFinder MyUsbFinder = new UsbDeviceFinder(1234, 1); #endregion public static void Main(string[] args) { ErrorCode ec = ErrorCode.None; try { // Find and open the usb device. MyUsbDevice = UsbDevice.OpenUsbDevice(MyUsbFinder); // If the device is open and ready if (MyUsbDevice == null) throw new Exception("Device Not Found."); // If this is a "whole" usb device (libusb-win32, linux libusb-1.0) // it exposes an IUsbDevice interface. If not (WinUSB) the // 'wholeUsbDevice' variable will be null indicating this is // an interface of a device; it does not require or support // configuration and interface selection. IUsbDevice wholeUsbDevice = MyUsbDevice as IUsbDevice; if (!ReferenceEquals(wholeUsbDevice, null)) { // This is a "whole" USB device. Before it can be used, // the desired configuration and interface must be selected. // Select config #1 wholeUsbDevice.SetConfiguration(1); // Claim interface #0. wholeUsbDevice.ClaimInterface(0); } // open read endpoint 1. UsbEndpointReader reader = MyUsbDevice.OpenEndpointReader(ReadEndpointID.Ep01); byte[] readBuffer = new byte[1024]; while (ec == ErrorCode.None) { int bytesRead; // If the device hasn't sent data in the last 5 seconds, // a timeout error (ec = IoTimedOut) will occur. ec = reader.Read(readBuffer, 5000, out bytesRead); if (bytesRead == 0) throw new Exception(string.Format("{0}:No more bytes!", ec)); Console.WriteLine("{0} bytes read", bytesRead); // Write that output to the console. Console.Write(Encoding.Default.GetString(readBuffer, 0, bytesRead)); } Console.WriteLine("\r\nDone!\r\n"); } catch (Exception ex) { Console.WriteLine(); Console.WriteLine((ec != ErrorCode.None ? ec + ":" : String.Empty) + ex.Message); } finally { if (MyUsbDevice != null) { if (MyUsbDevice.IsOpen) { // If this is a "whole" usb device (libusb-win32, linux libusb-1.0) // it exposes an IUsbDevice interface. If not (WinUSB) the // 'wholeUsbDevice' variable will be null indicating this is // an interface of a device; it does not require or support // configuration and interface selection. IUsbDevice wholeUsbDevice = MyUsbDevice as IUsbDevice; if (!ReferenceEquals(wholeUsbDevice, null)) { // Release interface #0. wholeUsbDevice.ReleaseInterface(0); } MyUsbDevice.Close(); } MyUsbDevice = null; // Free usb resources UsbDevice.Exit(); } // Wait for user input.. Console.ReadKey(); } } } }