How to read properties file placed outside war?
Solution 1
Provide the file name using context param or java system parameter.
1.Context Param
<context-param>
<param-name>daofilename</param-name>
<param-value>D:\daofilename.props</param-value>
</context-param>
2.System Parameter
java -Ddao.filename=D:\daofilename.props server
Solution 2
This is how I do this:
Add the following to context.xml file (conf folder, in tomcat installation dir). you may change the name attribute; and in value set the path of the folder where you have your properties files.
<Environment name="config" value="C:\Program files\my app\"
type="java.lang.String" override="false"/>
Then in your util class, you can get the file like this: (in "java:comp/env/config" replace 'config' with the value of the "name" attribute you used in context.xml)
String folderName = null;
Properties properties = new Properties();
try {
InitialContext context = new InitialContext();
folderName = (String) context.lookup("java:comp/env/config");
} catch (NamingException ex) {
System.out.println("exception in jndi lookup");
}
if(folderName != null) {
File configFile = new File(folderName + "yourfile.properties");
try {
InputStream is = new FileInputStream(configFile);
properties.load(is);
} catch(IOException ex) {
System.out.println("exception loading properties file");
}
}
Hope this helps you or anyone else.
Solution 3
Depending on your web server, you can place the properties file in some location that is included in the classpath. For example, for some tomcat versions, that would be ${TOMCAT_BASE}/shared/classes
. The webapp can then use something like the following to read the file and have it be automatically found in this location.
ServletContext context = getServletContext();
InputStream is = context.getResourceAsStream("yourfilename.cnf");
You can also name the file after your webapp's installed name and use that name in your code when loading the file from the classpath. That way, you can have the properties files for multiple webapps in the shared directory without conflicting with each other.
You've indicated that you don't have access to the ServletContext because you want the code to be in a utility class. One way you can get around this limitation is to register a ServletContextListener that creates an instance of your property file reader (since it has access to the context) and registers it so other code can use it. Something like the following:
public class MyServletContextListener extends ServletContextListener{
public void contextInitialized(ServletContextEvent event){
ServletContext context = event.getServletContext();
context.setAttribute("settings", new MyPropertyReader(context));
}
public void contextDestroyed(ServletContextEvent event){}
}
}
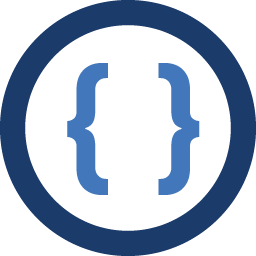
Admin
Updated on January 21, 2020Comments
-
Admin over 4 years
I'm working on a web application these days. That uses only jsps and servlets. It's a small application. Right now I have placed all the DataSource details in DAO utility class. I want to place these details in a properties file that can be placed outside the war, so that depending on the environment we can change these values, without effecting the war. How can I achieve this?
-
Gursel Koca almost 13 yearsBut, it is again property file location is war dependent. You should change war file to change propert file location.
-
Ramesh PVK almost 13 yearsIf i am correct the requirement was to change the props in the file , not the file as such.
-
Ramesh PVK almost 13 years@Gursel Koca If that was the case, Even the system parameter requires change in the run script file
-
Stout Joe over 7 yearsThis answer helped me so much. Don't forget to close your input streams, everyone.