How to redirect the output of print to a TXT file
Solution 1
If you're on Python 2.5 or earlier, open the file and then use the file object in your redirection:
log = open("c:\\goat.txt", "w")
print >>log, "test"
If you're on Python 2.6 or 2.7, you can use print as a function:
from __future__ import print_function
log = open("c:\\goat.txt", "w")
print("test", file = log)
If you're on Python 3.0 or later, then you can omit the future import.
If you want to globally redirect your print statements, you can set sys.stdout:
import sys
sys.stdout = open("c:\\goat.txt", "w")
print ("test sys.stdout")
Solution 2
To redirect output for all prints, you can do this:
import sys
with open('c:\\goat.txt', 'w') as f:
sys.stdout = f
print "test"
Solution 3
A slightly hackier way (that is different than the answers above, which are all valid) would be to just direct the output into a file via console.
So imagine you had main.py
if True:
print "hello world"
else:
print "goodbye world"
You can do
python main.py >> text.log
and then text.log will get all of the output.
This is handy if you already have a bunch of print statements and don't want to individually change them to print to a specific file. Just do it at the upper level and direct all prints to a file (only drawback is that you can only print to a single destination).
Solution 4
Building on previous answers, I think it's a perfect use case for doing it (simple) context manager style:
import sys
class StdoutRedirection:
"""Standard output redirection context manager"""
def __init__(self, path):
self._path = path
def __enter__(self):
sys.stdout = open(self._path, mode="w")
return self
def __exit__(self, exc_type, exc_val, exc_tb):
sys.stdout.close()
sys.stdout = sys.__stdout__
and then:
with StdoutRedirection("path/to/file"):
print("Hello world")
Also it would be really easy to add some functionality to StdoutRedirection
class (e.g. a method that lets you change the path)
Solution 5
Usinge the file
argument in the print
function, you can have different files per print:
print('Redirect output to file', file=open('/tmp/example.log', 'w'))
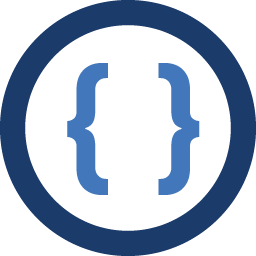
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
I have searched Google, Stack Overflow and my Python users guide and have not found a simple, workable answer for the question.
I created a file c:\goat.txt on a Windows 7 x64 machine and am attempting to print "test" to the file. I have tried the following based on examples provided on StackOverflow:
At this point I don't want to use the log module since I don't understand from the documentation of to create a simple log based upon a binary condition. Print is simple however how to redirect the output is not obvious.
A simple, clear example that I can enter into my interperter is the most helpful.
Also, any suggestions for informational sites are appreciated (NOT pydocs).
import sys print('test', file=open('C:\\goat.txt', 'w')) #fails print(arg, file=open('fname', 'w')) # above based upon this print>>destination, arg print>> C:\\goat.txt, "test" # Fails based upon the above