How to redraw grid of widgets in flutter.io after receiving new items from network?
You should call setState whenever you change data that affects what the build() function for a given State object returns:
https://docs.flutter.io/flutter/widgets/State/setState.html
For example:
setState(() {
_recipes.add(job);
});
If you do this, I'd recommend changing kPestoRecipes from being a global constant to being a private member variable of a State subclass. That will probably involve passing the list around from one widget to another rather than having all the widget refer to the global constant.
One other tip: when you get the HttpClientResponse, you should check the mounted
property of the State object. If mounted
is false, that means the widget has been removed from the tree and you might want to ignore the network response.
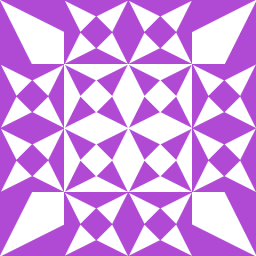
Moonwalker
Updated on December 01, 2022Comments
-
Moonwalker 21 days
I'm modifying Pesto example https://github.com/flutter/flutter/blob/76844e25da8806a3ece803f0e59420425e28a405/examples/flutter_gallery/lib/demo/pesto_demo.dart to receive new recipes from the net, by adding the following function:
void loadMore(BuildContext context, query) { HttpClient client = new HttpClient(); client.getUrl(Uri.parse("http://10.0.2.2:5000/search/" + query )) .then((HttpClientRequest request) { return request.close(); }) .then((HttpClientResponse response) { // Process the response. response.transform(UTF8.decoder).listen((contents) { // handle data var decoded = JSON.decode(contents); var rec = new Recipe( name: decoded['title'], author: decoded['author'], professionIconPath: 'images/1.png', description: decoded['description'], imageUrl: 'http://example.jpg', ); kPestoRecipes.add(job); //Navigator.push(context, new MaterialPageRoute<Null>( // settings: const RouteSettings(name: "/"), // builder: (BuildContext context) => new PestoHome(), //)); }); }); }
and I binded loading additional recipe to clicking interface button:
floatingActionButton: new FloatingActionButton( child: new Icon(Icons.edit), onPressed: () { scaffoldKey.currentState.showSnackBar(new SnackBar( content: new Text('Adding new recipe') )); loadMore(context, "test"); }, ),
But how do I redraw home page when new recipe received? I've tried to the part with Navigator you've seen commented out, but it didn't work. I also tried
new PestoDemo();
but it showed new recipe only for a moment and didn't feel like a proper way to do re-drawing.