How to remove drawer icon from appbar when the screen size is large and display it when the screen size is small | Flutter Web
344
you can use responsive_builder.
class NavBar extends StatelessWidget {
const NavBar({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return ScreenTypeLayout(
mobile: NavBarMobile(), // this will automatic take screensize and show the Nav bar accordingly
tablet: NavBarDesktop(),
);
}
}
in NavBarDesktop()
Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
Text("Logo Here"),
Row(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.center,
children: [
TextButton(
onPressed: () {},
child: Row(
children: [
Icon(
Icons.person,
color: Colors.white,
),
Text(
" About Me",
style: TextStyle(color: Colors.white),
)
],
),
style: ButtonStyle(padding: MaterialStateProperty.all<EdgeInsets>(EdgeInsets.all(20))),
),
TextButton(
onPressed: () {},
child: Row(
children: [
Icon(
Icons.cycle,
color: Colors.white,
),
Text(
" Blog",
style: TextStyle(color: Colors.white),
)
],
),
style: ButtonStyle(padding: MaterialStateProperty.all<EdgeInsets>(EdgeInsets.all(20))),
),
TextButton(
onPressed: () {},
child: Row(
children: [
Icon(
Icons.cycle,
color: Colors.white,
),
Text(
" Contact Me",
style: TextStyle(color: Colors.white),
)
],
),
style: ButtonStyle(padding: MaterialStateProperty.all<EdgeInsets>(EdgeInsets.all(20))),
),
],
),
],
),
and in NavBarMobile()
Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
OutlineButton(
onPressed: () {
Scaffold.of(context).openEndDrawer();
},
hoverColor: Colors.black,
child: Text("Menu"),
),
Text("Logo Here")
],
),
and Drawer
:
Drawer(
child: ListView(
// Important: Remove any padding from the ListView.
padding: EdgeInsets.zero,
children: <Widget>[
DrawerHeader(
decoration: BoxDecoration(
color: Colors.blue,
),
child: Text('Drawer Header'),
),
ListTile(
title: Text('About Me'),
onTap: () {
// Update the state of the app.
// ...
},
),
ListTile(
title: Text('Blog'),
onTap: () {
// Update the state of the app.
// ...
},
),
ListTile(
title: Text('Contact Me'),
onTap: () {
// Update the state of the app.
// ...
},
),
],
),
);
In scaffold
add this line so that if user slide from right than Drawer do not open in web
endDrawerEnableOpenDragGesture: false,
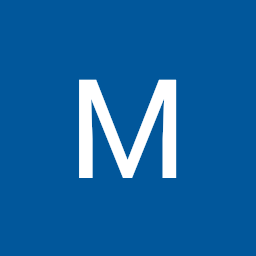
Author by
MURALI KRISHNAN MT
Updated on December 30, 2022Comments
-
MURALI KRISHNAN MT over 1 year
I'm creating a flutter web app, i have some option in top of the page such as about, contact. My problem is i want to show these option in Appbar when displaying in large screen and for mobile i want to put it inside drawer. I implement it using the below code, but the drawer icon is always there in large and small screens. I don't want to show it in large screen.
import 'package:flutter/material.dart'; class HomePage extends StatelessWidget { const HomePage({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Home"), ), drawer: LayoutBuilder( builder: (context, constraints) { if (constraints.maxWidth < 768) { return Drawer(); } else { return SizedBox(); } }, ), ); } }
How can i Implement this in correct way