How to Remove the First and Last double quotes in the JSON String in JavaScript?
10,880
Solution 1
I refactored your code so you can have a look how to do this getting rid of all these string operations:
If you work with objects- just work with them and serialize to JSON when you've got what you need:
fiddle: https://jsfiddle.net/myppwgmb/
And here is how I would have done it:
var sys = {
timestamp: "100",
seq: "5",
serviceid: "21",
srcip: "192.168.0.1",
ver: "1.0",
};
var hdr = {
appcontext: "context",
appname: "appName",
appid: "identifier",
cmd: "command",
timeout: "500",
option: "optionCommand",
};
var data = {
CntrlID: "6",
Devinst: "1",
btnstatus: "btnStatus",
DevID: "33",
value: "true",
status: "errorStatus",
}
var dataFinal = {
app: {
data: data,
hdr: hdr,
},
sys: sys
}
alert(JSON.stringify(dataFinal));
fiddle: https://jsfiddle.net/myppwgmb/1/
Solution 2
Here you go
var yourString = "{JsonString}";
var result = yourString.substring(1, yourString.length-1);
Or you can use .slice
var result = yourString.slice(1, -1);
Related videos on Youtube
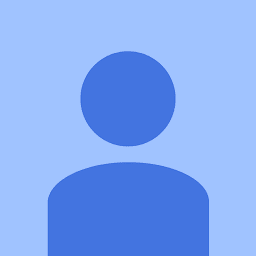
Author by
vinod chowdary
Updated on October 02, 2022Comments
-
vinod chowdary over 1 year
Hi guys I wanted to remove the double quotes from the first and last places of the JSON string and I need to place one JSON object in another and I will display my code and my requirement.
var sysMsg = new Object(); sysMsg.timestamp = "100"; sysMsg.seq = "5"; sysMsg.serviceid = "21"; sysMsg.srcip = "192.168.0.1"; sysMsg.ver = "1.0"; var sysString= JSON.stringify(sysMsg); window.alert(sysString); var systmMsg = new Object(); systmMsg.sys = sysString; // JSON.stringify(hdrString); var sysFinal= JSON.parse(systmMsg); alert(sysFinal); sysFinal = sysFinal.replace(/\\/g, ""); window.alert(sysFinal); var hdrMsg = new Object(); hdrMsg.appcontext = "context"; hdrMsg.appname = "appName"; hdrMsg.appid = "identifier"; hdrMsg.cmd = "command"; hdrMsg.timeout = "500"; hdrMsg.option = "optionCommand"; var hdrString= JSON.stringify(hdrMsg); //window.alert(hdrString); var hdrFinal = new Object(); hdrFinal.hdr = hdrString; var hdrFinalMsg= JSON.stringify(hdrFinal); hdrFinalMsg = hdrFinalMsg.replace(/\\/g, ""); window.alert(hdrFinalMsg); var dataMsg = new Object(); dataMsg.CntrlID = "6"; dataMsg.Devinst = "1"; dataMsg.btnstatus = "btnStatus"; dataMsg.DevID = "33"; dataMsg.value = "true"; dataMsg.status = "errorStatus"; var dataString = JSON.stringify(dataMsg); //window.alert(dataString); var dataFinal = new Object(); dataFinal.data = dataString; var dataFinalMsg= JSON.stringify(dataFinal); dataFinalMsg = dataFinalMsg.replace(/\\/g, ""); window.alert(dataFinalMsg); var appData = hdrFinalMsg.concat(dataFinalMsg); var appFinal = new Object(); appFinal.app = JSON.stringify(appData); var appFinalMsg= JSON.stringify(appFinal); appFinalMsg = appFinalMsg.replace(/\\/g, ""); window.alert(appFinalMsg); var finalData = appFinalMsg.concat(sysFinal); var finalMsg= JSON.stringify(finalData); finalMsg = finalMsg.replace(/\\/g, ""); window.alert(finalMsg);
Here I am getting the string as following
"{"app":""{"hdr":"{"appcontext":"context","appname":"appName","appid":"identifier","cmd":"command","timeout":"500","option":"optionCommand"}"}{"data":"{"CntrlID":"6","Devinst":"1","btnstatus":"btnStatus","DevID":"33","value":"true","status":"errorStatus"}"}""}{"sys":"{"timestamp":"100","seq":"5","serviceid":"21","srcip":"192.168.0.1","ver":"1.0"}"}"
but I want the output as below
{"app":{"data":{"Cntrl.ID":"6","Dev.inst":"1","btn.status":"btnStatus","Dev.ID":"33","value":"true","status":"errorStatus"},"hdr":{"appcontext":"context","appname":"appName","appid":"identifier","cmd":"set","timeout":"500","option":"optionCommand"}},"sys":{"srcip":"192.168.0.1","ver":"1.0","serviceid":"21","seq":"5","timestamp":"100"}}
-
vinod chowdary over 7 yearsThank you very very much sir.. for your help, Incase if I need to get the data in the array dynamically how can I do at that point of situation.Thanks in advance
-
Wolfgang over 7 yearsso.. what does your array look like? You havn't specified one right now.
-
vinod chowdary over 7 yearsI have to get the data ctrlID DevID and all from another file and I need to update them each time. so I asked you how to update the values dynamically
-
Wolfgang over 7 years@vinod chowdary if you provide a fiddle I'm happy to help
-
Urasquirrel almost 5 yearsWhile fixing this problem we invalidated the question for any other users... Should rename the question.