How to replace JPanel with another JPanel
22,674
Solution 1
don't to use
AbsoluteLayout
change
validate();
inactionPerformed
tocontain.validate();
and follows withcontain.repaint();
rename class name (reserved Java word, or methods name)
Frame
(java.awt.Frame
) toMyFrame
(for example)use
CardLayout
instead of remove and then add a newJPanel
on runtime
Solution 2
Try this, Following code will load the second jPanel (NewOrder) to first jPanel(jpMain)
NewOrder no = new NewOrder(); //This is the object of Second JPanel
// jpMain - This is the First JPanel
jpMain.setLayout(new java.awt.BorderLayout());
jpMain.removeAll();
jpMain.add(no);
jpMain.revalidate();
Solution 3
You must call validate()
and then repaint()
on the containing panel after you do the remove and add operations.
contain.validate();
contain.repaint();
Solution 4
you need to do like this :
public void actionPerformed(ActionEvent e) {
//System.out.println("in");
contain = getContentPane();
contain.removeAll();
//System.out.println("in2");
reChange2 = new JPanel(null);
reChange2.setBackground(Color.white);
reChange2.setSize(240, 225);
reChange2.setBounds(50, 50, 240, 225);
//System.out.println("in3");
contain.add(reChange2);
validate();
repaint();
//System.out.println("in4");
setVisible(true);
//System.out.println("in5");
}
});
Solution 5
Several issues with your code. Here is fixed version:
public class Frame extends JFrame {
private Container contain;
private JPanel reChange,reChange2;
private JButton reChangeButton;
public Frame() {
super("Change a panel");
setSize(350, 350);
getContentPane().setLayout(null); // Changed here
setLocationRelativeTo(null);
setResizable(false);
reChange = new JPanel(null);
reChange.setBackground(Color.red);
reChange.setSize(240, 225);
reChange.setBounds(50, 50, 240, 225);
getContentPane().add(reChange); // Changed here
reChangeButton = new JButton("Change It");
reChangeButton.setBounds(20, 20, 100, 20);
getContentPane().add(reChangeButton); // Changed here
reChangeButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
contain = getContentPane();
contain.removeAll();
reChange2 = new JPanel(null);
reChange2.setBackground(Color.white);
reChange2.setSize(240, 225);
reChange2.setBounds(50, 50, 240, 225);
contain.add(reChange2);
invalidate(); // Changed here
repaint(); // Changed here
}
});
}
public static void main(String[] args) {
Frame frame = new Frame();
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
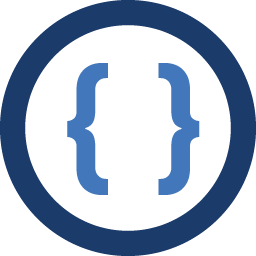
Author by
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I want to replace a Jpanel with another one in a JFrame I already search and try my code but nothing's happen this is my code :
public class Frame extends JFrame { private Container contain; private JPanel reChange,reChange2; private JButton reChangeButton; public Frame() { super("Change a panel"); setSize(350, 350); setLayout(null); setLocationRelativeTo(null); setResizable(false); reChange = new JPanel(null); reChange.setBackground(Color.red); reChange.setSize(240, 225); reChange.setBounds(50, 50, 240, 225); add(reChange); reChangeButton = new JButton("Change It"); reChangeButton.setBounds(20, 20, 100, 20); add(reChangeButton); reChangeButton.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { //System.out.println("in"); contain = getContentPane(); contain.removeAll(); //System.out.println("in2"); reChange2 = new JPanel(null); reChange2.setBackground(Color.white); reChange2.setSize(240, 225); reChange2.setBounds(50, 50, 240, 225); //System.out.println("in3"); contain.add(reChange2); validate(); //System.out.println("in4"); setVisible(true); //System.out.println("in5"); } }); } public static void main(String[] args) { Frame frame = new Frame(); frame.setVisible(true); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } }
can someone help me ? Thanks a lot