How to reset/clear file Input
Solution 1
You can use ref
to reset the file uploader value.
this.$refs.fileupload.value = null;
codepen - https://codepen.io/Pratik__007/pen/MWYVjqP?editors=1010
Solution 2
I tried several suggestions I found on Stackoverflow, but in my project there were several errors. What solved my problem was:
<input type="file" ref="inputFile"/>
this.$refs.inputFile.reset();
This code above resets the field values.
Solution 3
To reset the input, I let vue rerender it. Just add a key to the input and change the value of the key whenever you want the file input to be cleared.
Like so:
window.app = new Vue({
el: '#app',
data: {
fileInputKey: 0
},
methods:{
inputChange() {
console.log('files chosen');
},
clear() {
this.fileInputKey++;
}
}
});
<script src="https://cdnjs.cloudflare.com/ajax/libs/vue/2.5.17/vue.js"></script>
<div id="app">
<input type="file" @change="inputChange($event)" :key="fileInputKey"/>
<button @click="clear">Clear</button>
</div>
Solution 4
I also came to this problem and could not reset the value. after going through the code of all previous projects and modifying them I got a lot of methods through which a file input could be reset. one of the way is do is to capture the event object if you want to perform it after handling some code. another way is to change the type of the input element and change it back again.
<div id="app">
<input type="file" @change="onFilePicked" ref="file">
<button @click="clear()">Cancel</button>
</div>
var v = new Vue({
el:'#app',
data:{},
methods:{
clear() {
this.$refs.file.type='text';
this.$refs.file.type='file';
},
onFilePicked(event){
//if you directly want to clear the file input after handling
//some code........
event.target.value=null;
//OR
event.target.value='';
}
},
});
Solution 5
For Composition api
<input type="file"
class="custom-file-input"
id="customFileLang"
lang="en"
accept="image/*"
:class="{ 'is-invalid': errors && errors.images }"
@change="previewImg" multiple
>
Inside setup function:
const imgInput = ref(null) // for input type file
const previewImg = (event) => {
imgInput.value = event
// rest of code to preview img
}
const uploadImage = () => {
//on success
imgInput.value.target.value = null //reset input type file
}
Related videos on Youtube
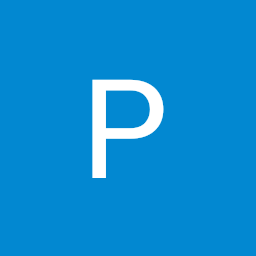
Peter Parker
Updated on September 24, 2021Comments
-
Peter Parker over 2 years
I am stuck with this problem from resetting an image file from input type=file. This is the scenario, I set an image and then I clicked the 'Cancel' button (which means it was reset), and then I will set again the same image, it will not set. I do not know why but I think it is a bug.
Here is my code for resetting the image/form.
resetImage () { this.$refs.addImageForm.reset() this.dialog = '' this.imgSrc = '' this.fileName = '' this.imgUrl = '' this.file = '' }
I am using Vue.js and Vuetify if that helps. I hope you can help me. I am stuck with this problem
HERE IS THE HTML
<template> <v-dialog persistent v-model="dialog" width="600" > <template v-slot:activator="{ on }"> <v-btn depressed color="primary" v-on="on"> <v-icon class="pr-2">check</v-icon>Approve </v-btn> </template> <v-card> <div class="px-4 pt-3"> <span class="subheading font-weight-bold">Approve Details</span> <input ref="image" type="file" name="image" accept="image/*" style="display: none;" @change="setImage" /> <v-layout row wrap align-center> <v-flex xs12 class="pa-0"> <div class="text-xs-center"> <v-img :src="imgUrl" contain/> </div> <VueCropper :dragMode="'none'" :viewMode="1" :autoCrop="false" :zoomOnWheel="false" :background="false" :src="imgSrc" v-show="false" ref="cropper" /> <v-form ref="addImageForm" lazy-validation> <v-layout row wrap class="pt-2"> <v-flex xs12> <v-text-field outline readonly :label="imgSrc ? 'Click here to change the image' : 'Click here to upload image'" append-icon='attach_file' :rules="imageRule" v-model='fileName' @click='launchFilePicker' ></v-text-field> </v-flex> </v-layout> </v-form> </v-flex> </v-layout> </div> <v-divider></v-divider> <v-card-actions class="px-4"> <v-btn large flat @click="resetImage()" >Cancel</v-btn> <v-spacer></v-spacer> <v-btn large depressed color="primary" @click="approveCashout" :loading="isUploading" >Approve</v-btn> </v-card-actions> </v-card> </v-dialog> </template>
-
haMzox over 4 yearsCan you show your HTML as well?
-
Peter Parker over 4 yearsSure2 wait I will edit
-
Peter Parker over 4 yearsUpdated and added html
-
-
Abdullah Al Mamun almost 4 yearsTnx a lot. You save my day.
-
Patel Pratik almost 4 yearsI'm glad to hear it :)
-
OWADVL over 3 yearsthe above answer throws some warning. this one should be the accepted answer
-
mankowitz over 3 yearsI get
TypeError: this.$refs.inputFile.reset is not a function
when I use this, but usingthis.$refs.inputFile.value=null
worked for me. -
Zapnuk over 3 yearsIn my setup - Vue 2.6.12, Vuetify 2.4.2, Typescript 3.9.7 this results in a 'Vue warning': "Avoid mutating a prop directly since the value will be overwritten whenever the parent component re-renders. [...]" It still works, but so does the answer below (besides vs code reporting a nonexistent error)
-
Mohamed Raza almost 3 yearsthis throws an error
Cannot set property 'value' of undefined"
-
Patel Pratik almost 3 years@MohamedRaza Make sure you have added ref in input field
-
Mohamed Raza almost 3 years@PatelPratik i already added ref in my input
-
Patel Pratik almost 3 years@MohamedRaza Can you try to console log this.$refs.fileupload and check it's giving value or not
-
Ahmed Aboud almost 3 yearsthis.$refs.inputFile.$el.value=null is better
-
Sigit over 2 yearswork like a charm, this is the right way how to reset the file input form on vuetify. Thanks
-
josevoid over 2 yearsFor
this.$refs.fileupload.value=null;
to work, you need to assign ref on the input as well like this<input ref="fileupload" type="file" />
. -
Patel Pratik over 2 yearsYup it's required. It's there in codepen and question.
-
Lema about 2 yearsWorks like charm and no warning, Vue.js 2.6.11
-
Lema about 2 yearsThis does not work for me, says reset is not a function and removing brackets doesn't do anything. Neither warning nor error and nothing happens, Vue.js 2.6.11
-
Niels almost 2 yearsOf all the solutions, this was the only one that works :)