How to return a list of objects in ASP.NET Web API async controller method
28,840
Solution 1
Never return a Task
from an action method if you are not executing anything async
: it is useless and will also adds unnecessary overhead to your call.
To return a list of objects, simply declare the correct return type:
[HttpGet("[action]/{accountNumber}")]
public List<SubscriberHistory> GetSubscriberHistory(string accountNumber)
{
SubscriberManager subsManager = new SubscriberManager();
return subsManager.GetSubscriberHistoryByAccountNumber(accountNumber);
}
If you, instead, want to do it asynchronously, then you'll have to return a Task<T>
and await the result:
[HttpGet("[action]/{accountNumber}")]
public async Task<List<SubscriberHistory>> GetSubscriberHistory(string accountNumber)
{
SubscriberManager subsManager = new SubscriberManager();
return await subsManager.GetSubscriberHistoryByAccountNumber(accountNumber);
}
And in your manager:
public async Task<List<SubscriberHistory>> GetSubscriberHistory()
{
List<SubscriberHistory> list = new List<SubscriberHistory>();
var result = await client.GetAsync("http://myhost/mypath");
//Your code here
return list;
}
Solution 2
If you want to use Async Controller, you need to update your code like this:
[HttpGet("[action]/{accountNumber}")]
public async Task<IHttpActionResult> GetSubscriberHistory(string accountNumber)
{
SubscriberManager subsManager = new SubscriberManager();
return await subsManager.GetSubscriberHistoryByAccountNumber(accountNumber);
}
There should be async and await in your Controller function if you want to use Async controller. Thanks!
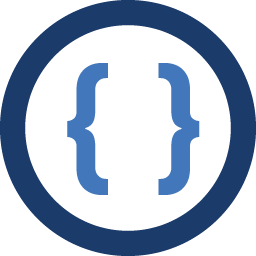
Author by
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
How to return a list of objects in the ASP.NET Web API async controller method or wrap a list inside the Task object?
Class:
public class SubscriberHistory { public string Date; public string Message; public SubscriberHistory(string Date, string Message) { this.Date = Date; this.Message = Message; } }
Controller:
[HttpGet("[action]/{accountNumber}")] public Task<SubscriberHistory> GetSubscriberHistory(string accountNumber) { SubscriberManager subsManager = new SubscriberManager(); return subsManager.GetSubscriberHistoryByAccountNumber(accountNumber); }
Controller Manager:
public async List<SubscriberHistory> GetSubscriberHistory() { List<SubscriberHistory> List = new List<SubscriberHistory>(); // HttpClient get data return List; // ? }
-
Admin almost 8 yearsThanks, Federico! Inside of my method I'm trying to get data from a web service and to do that I have to use the HttpClient class, which in turn uses asynchronous methods
-
Federico Dipuma almost 8 yearsI added the asynchronous approach inside the answer. Always remember that to be able to use
async
/await
your entire call chain must becomeasync
and return aTask
/Task<T>
. -
Admin almost 8 yearsGreat! Thank you Federico. That's what i need.