How to return a value with Dispatcher.Invoke?
Solution 1
There's another way that returns value from Invoke():
object oIsLoaded = container.Dispatcher.Invoke( new Func<bool> ( () =>
{
return container.IsLoaded;
})
);
And by the way, chances are that the initial code (which is working with delegate) won't modify oIsLoaded
at all; So I'd rather use a Func<>
for returning a value from that kind of function.
Solution 2
This is my method to retrieve selected value for a combobox, how can I say delegate to return value?
private object getValueCB(System.Windows.Controls.ComboBox cb)
{
object obj;
if (!cb.Dispatcher.CheckAccess())
{
obj = cb.Dispatcher.Invoke(
System.Windows.Threading.DispatcherPriority.Normal,
new Action(
delegate()
{
obj = cb.SelectedValue;
}
));
return obj;
}
else
{
return obj = cb.SelectedValue;
}
}
Solution 3
I have solved this. The solution is create a custom delegate that returns the desired type like this:
private object GetValueCB(System.Windows.Controls.ComboBox cb)
{
object obj = null;
if (!cb.Dispatcher.CheckAccess())
{
obj = cb.Dispatcher.Invoke(
System.Windows.Threading.DispatcherPriority.Normal,
(MyDelegate)
delegate()
{
return (obj = cb.SelectedValue);
}
);
return obj;
}
else
{
return obj = cb.SelectedValue;
}
}
public delegate object MyDelegate();
Solution 4
You can't do this directly but you can do this.
Dispatcher.Invoke() actually returns the return value from the delegate you call, so alter your delegate accordingly.
Return Value
Type: System.Object The return value from the delegate being invoked or null if the delegate has no return value.
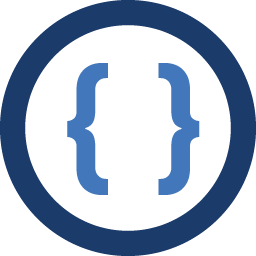
Admin
Updated on June 15, 2020Comments
-
Admin almost 4 years
Anyone knows how to return a value from
Dispatcher
.Invoke
in wpf? I want to return the selected index for a ComboBox.Thanks!
-
Admin about 14 yearsAbove I post my snippet code, how could I modify this to allow delegate returns selected value for combobox? thanks
-
Chris about 14 yearsAction does not allow a return value, in this case you will have to use a solution like @Will
-
Admin about 14 yearsWill solution doesn't work in ComboBox WPF Control. I get an error.