How to return the correct content-type for JSON in CakePHP?
Solution 1
I make Ajax calls to retrieve JSON content in all of my projects and I've never done most of what you're doing here. The extent of my controller code looks something like this:
public function do_something_ajaxy() {
Configure::write ( 'debug', 0 );
$this->autoRender = false;
/** Business logic as required */
echo json_encode ( $whatever_should_be_encoded );
}
I make my Ajax calls via jQuery so I suppose that could make a difference, but it would surprise me. In this case, you're problem appears to be in the handler, not with the caller. I'd recommend removing lines 17-23 and replacing them with a simple echo json_encode ( array('response' => $actions[0]) )
statement.
You're also testing for $this->RequestHandler->isGet()
. Try testing $this->RequestHandler->isAjax()
instead. I'm not sure whether Ajax calls are recognized as by both their type and their method.
Solution 2
After reading this and this, I got the following to return "Content-Type:application/json":
Configure::write('debug', 0);
$this->RequestHandler->respondAs('json');
$this->autoRender = false;
echo json_encode($data);
With JQuery's $.getJSON method, I'm still getting
Resource interpreted as image but transferred with MIME type text/html.
But at least my data is parsing.
Solution 3
I've also just had this problem, and solved it by using:
$this->RequestHandler->respondAs('text/x-json');
Also make sure that "debug" in your config file is set to less than 2 otherwise the header will not be set.
Solution 4
I am not sure (and, to be honest, I've never used CakePHP), but you may want to try to specify a second argument in the setContent method..
replace this:
$this->RequestHandler->setContent('json')
with this:
$this->RequestHandler->setContent('json', 'text/x-json');
see this file for an example..
Solution 5
I've been having the same problem as the original poster, and what worked for me was to follow Rob Wilkerson's advice, but also make sure I was using
jQuery.ajax()
instead of
jQuery.get()
or
jQuery.post()
jQuery.ajax() allows you to set the dataType to 'json' whereas the other two don't seem to allow you to set the datatype at all. When I set the data type in the AJAX request to 'json' it all worked as it should.
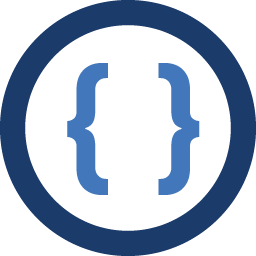
Admin
Updated on June 12, 2022Comments
-
Admin almost 2 years
I'm trying to set the content-type header for a JSON response accessed with an AJAX GET request. I've followed tutorials on blogs and the bakery but I always receive 'text/html' back from CakePHP. How do I set the content-type header correctly?
Here's my code at the moment:
public function admin_controller_actions_ajax() { Configure::write('debug', 0); if ($this->RequestHandler->isGet()) { $this->autoRender = FALSE; $aco_id = $this->params['url']['aco_id']; $aro_id = $this->params['url']['aro_id']; assert('$aco_id != NULL && $aro_id != NULL && is_numeric($aco_id) && is_numeric($aro_id)'); $actions = $this->Resource->getActionsForController($aco_id, $aro_id); assert('is_array($actions) && is_array($actions[0])'); /* I made RequestHandler part of the $components property */ $this->RequestHandler->setContent('json'); $this->RequestHandler->respondAs('json'); /* I've tried 'json', 'JSON', 'application/json' but none of them work */ $this->set('json_content', json_encode(array('response' => $actions[0]))); $this->layout = NULL; $this->render('/json/default'); } } /* json/default.ctp */ <?php echo $json_content; ?>
Any help would be appreciated.
Thanks,
-- Isaac