How to run a .class file that is part of a package from cmd?
Solution 1
Suppose you did cd C:/projects
and HelloWorld.class
is in C:/projects/com
, then just type:
java com.HelloWorld
Solution 2
Packages are directly related to the expected directory location of the file.
That is, if you have a source file with the package directive of com
, it is expected that the file will live in the com
directory.
In your HelloWorld
example, it would be expected that the HelloWorld.java
file would be stored in the com
directory, like com\HelloWorld.java
When you compile the file, it will create a class file called HelloWorld.class
in the com
directory, like com\HelloWorld.class
This way, when Java goes looking for the com.HelloWorld
class, it would actually be searching it's class path and appending com\HelloWorld.class
to it until it finds your class file or runs out of class path
Example
So, I copied your HelloWorld.java
(with package) example to C:\java\com\HelloWord.java
From the command line, I changed to the C:\java
directory...
C:\java>dir com
Volume in drive C is OS
Volume Serial Number is ####-####
Directory of C:\java\com
09/08/2013 01:55 PM <DIR> .
09/08/2013 01:55 PM <DIR> ..
09/08/2013 01:55 PM 135 HelloWorld.java
Then I compiled the HelloWorld.java
C:\java>javac com\HelloWorld.java
Then I ran it...
C:\java>java com.HelloWorld
Hello World!
You might like to have a read through Packages tutorial
Solution 3
The syntax is:
java -classpath /path/to/package-folder <packageName>.<MainClassName>
So you may try: java com.HelloWorld
which would expect com/HelloWorld.class
file to be present as classpath by default points to the current directory (if not specified).
In case you're in different folder, try specifying classpath:
$ CLASSPATH=/path/to/package-folder/ java com.HelloWorld
Hello World!
$ java -cp /path/to/package-folder/ com.HelloWorld
Hello World!
$ cd /path/to/package-folder/ && java com.HelloWorld
Hello World!
For further explanation, check: How do I run Java .class files?
Solution 4
Run the program from the parent directory of the com directory.
java com.HelloWorld
Solution 5
Suppose the file locates at C:/projects/com/HelloWorld
and you can try the following ways.
1.java -cp c:/projects com.HelloWorld
2.cd c:/projects
java com.HelloWorld
(The example 2 is not working in many situation,such as java process.)
if there is no package declaration and there will be a little change.
1.java -cp c:/projects/com HelloWorld
2.cd c:/projects/com
java HelloWorld
(It's not good with the same reason.)
alternatively,relative path will be ok but has some risk. Last remember put the class file in end of cmd.
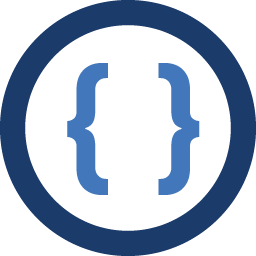
Admin
Updated on July 29, 2022Comments
-
Admin almost 2 years
I keep getting errors when I make my
.class
part of apackage
and try to run it from cmd.Here's the code that works after using
javac
and then java:class HelloWorld { public static void main(String[] args) { System.out.println("Hello World!"); } }
and then the code that does not work:
package com; class HelloWorld { public static void main(String[] args) { System.out.println("Hello World!"); } }
giving me this error after trying to run the program via the command:
java HelloWorld
:Exception in thread "main" java.lang.NoClassDefFoundError: HelloWorld (wrong nam e: com/HelloWorld) at java.lang.ClassLoader.defineClass1(Native Method) at java.lang.ClassLoader.defineClass(Unknown Source) at java.security.SecureClassLoader.defineClass(Unknown Source) at java.net.URLClassLoader.defineClass(Unknown Source) at java.net.URLClassLoader.access$100(Unknown Source) at java.net.URLClassLoader$1.run(Unknown Source) at java.net.URLClassLoader$1.run(Unknown Source) at java.security.AccessController.doPrivileged(Native Method) at java.net.URLClassLoader.findClass(Unknown Source) at java.lang.ClassLoader.loadClass(Unknown Source) at sun.misc.Launcher$AppClassLoader.loadClass(Unknown Source) at java.lang.ClassLoader.loadClass(Unknown Source) at sun.launcher.LauncherHelper.checkAndLoadMain(Unknown Source)
Here's what I've tried doing so far:
java -cp . HelloWorld java -cp . com.HelloWorld java -cp . com/HelloWorld java HelloWorld java com.HelloWorld java com/HelloWorld
Keep in mind that
javac
returns with no errors and that simply removingpackage com;
solves the problem. Sometimes in other scenarios I get an error that says the main class file cannot be found or something along those lines.What am I doing wrong?
-
Admin over 10 yearsThe file now resides in C:\com. When I try out your command it says:
-
Admin over 10 yearsError: COuld not find or load main class com.HelloWorld
-
sgbj over 10 yearsAt that point you must be cd'd into C:\, not C:\com. In other words, you need to try running it from outside of the package directory.
-
Admin over 10 yearsThe file now lives in C:\com. The problem is the same when I use "java HelloWorld" and when I try "java com.HelloWorld" like @sbat suggested it cannot find or load the main class.
-
MadProgrammer over 10 yearsOkay. Did you try my example? You could replace
C:\java\com
forC:\com
because, as I demonstrated, it works fine... -
user763410 over 8 yearsthere is a typo. You meant to say live but said life
-
Anand Builders almost 7 yearsBut why should one run from outside. How can one run from inside?
-
Combine about 5 yearsFor me worked: javac -d HelloWord.java and then -> java com.HelloWorld