How to run my winform application when computer starts
You can add a shortcut to your application in the startup folder, or through a registry value (Most applications use the HKLM or HKCU\Software\Microsoft\Windows\CurrentVersion\Run
key in the registry or put a shortcut in the C:\Users\<user name>\AppData\Roaming\Microsoft\Windows\Start Menu\Programs\Startup
folder. There are other options but these are the most popular)
Sample:
Microsoft.Win32;
...
//Startup registry key and value
private static readonly string StartupKey = "SOFTWARE\\Microsoft\\Windows\\CurrentVersion\\Run";
private static readonly string StartupValue = "MyApplicationName";
...
private static void SetStartup()
{
//Set the application to run at startup
RegistryKey key = Registry.CurrentUser.OpenSubKey (StartupKey, true);
key.SetValue(StartupValue, Application.ExecutablePath.ToString());
}
You can see the results of this code in regedit
:
The Run
key (And alternatively the RunOnce
key for running your application once) will run all applications that are in it on startup/when a user logs on.
This is the code I use in my applications, and it works great. You don't need any special installer to do this, you can simply call this method every time your app starts and it will set/update the applications value in the Run
key in the registry with the path to the executable.
The startup folder alternative is a little more involved to set up, check out this tutorial for help.
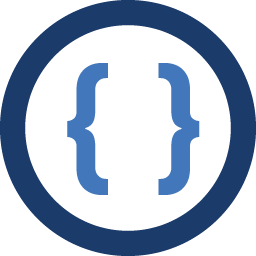
Admin
Updated on June 28, 2022Comments
-
Admin almost 2 years
I am just trying to run my winform application when computer starts. I have made the taskbar icon to appear on the left hand side of the system tray. My function everything is working well. But I need in such a way that if I install the winform in a computer. I need this to run after the computer restarts manually or automatically.
For now its like if I restart the application I again need to launch the application to run it. But I need something like to atuomatically launch the application on system restart. Any idea.
Codes i am trying
private void Form1_Load(object sender, EventArgs e) { timer1.Start(); notifyIcon1.BalloonTipTitle = "Minimize to Tray App"; notifyIcon1.BalloonTipText = "You have successfully minimized your form."; notifyIcon1.Visible = true; notifyIcon1.ShowBalloonTip(100); this.WindowState = FormWindowState.Minimized; this.ShowInTaskbar = false; } private void notifyIcon1_MouseDoubleClick(object sender, MouseEventArgs e) { this.Show(); this.WindowState = FormWindowState.Normal; this.ShowInTaskbar = true; } private void exitToolStripMenuItem_Click(object sender, EventArgs e) { System.Environment.Exit(0); }
-
Admin over 9 yearsi didnt get any thing.. I understood the article i found in codeproject its just making a shortcut in the same directory. I need something to automatically.. run my application on Windows STARTUP... not creating a shortcut..
-
Admin over 9 yearsALso i am getting an error called
"The type or namespace name 'Win32' does not exist in the namespace 'System' "
-
Cyral over 9 yearsIf you create a shortcut in the users Startup folder it will work. To solve the error you are seeing, include the reference to Win32 by right clicking the project > Add reference and finding it.
-
Cyral over 9 yearsNo problem, glad I could help!
-
Admin over 9 yearsSo when i gave the namespace
using Microsoft.Win32;
It worked... thanks a lot -
Admin over 9 years
private void timer1_Tick(object sender, EventArgs e) { System.IO.DirectoryInfo dir = new System.IO.DirectoryInfo(@"path"); int count = dir.GetFiles().Length; System.IO.DirectoryInfo dir1 = new System.IO.DirectoryInfo(@"path"); int count2 = dir1.GetFiles().Length; if (count != count2) {taskbarNotifier2.Show("Folder Updates", "New files got copied in the Draft folder. Please Update!!", 500, 3000, 500); } else { ?? }}
-
Admin over 9 yearswhat i should give in else statement not to invoke this.. because by default it is running the whole time. I need to make ideal the winform this.. time what to do..
-
Cyral over 9 yearsWhat do you mean with the timer?
-
Admin over 9 yearstimer is a function that your winform application will tick each sec's or minutes or hours.. so i have kept my function in a timer such a way that.. i need to compare the both directory.. in 1 hour each interval of time