how to save array object data in redux store
Solution 1
First of all, in your reducer you don't need to use ...state
spread operator, since hives seems to be the only one variable in your state there. And second, you are iterating over each element of hives, therefore you are inputting them one by one thus overwriting the previous one. You are not appending it to array. Here's how you need to change your action:
export const getHives = () => {
return dispatch => {
axios.get(GET_HIVE_URL, HEADER).then(res => {
const status = res.data.status;
const hives = res.data.hives;
if (status == 'hiveFound') {
dispatch(setHives(hives));
}
});
};
};
This way it will write the whole array into that variable in redux.
Solution 2
You can try this below so you can store the whole array. assuming you already have the actions.
InitialState
export default {
hives:[]
}
HivesReducer
export default function counter(state = initialState.hives, action) {
switch (action.type) {
case Types.SET_HIVES:
return [...state, action.payload];
default:
return state;
}
}
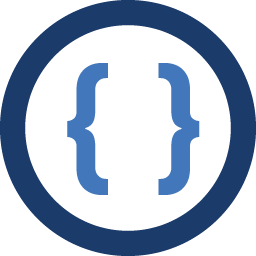
Admin
Updated on June 09, 2022Comments
-
Admin almost 2 years
i try to store multiple object in redux store on my react native app, but only one object is save, i'm new at redux, i try a lot of solutions found on StackOverflow but no one works :/
result i have in my store:
"hives": {"hive_id": 12944, "hive_name": null}
result i want (or something like that) :
"hives": [ 1: {"hive_id": 123, "hive_name": "HelloHive"}, 2: {"hive_id": 12944, "hive_name": null}]
store:
const middleware = [thunk] export const store = createStore(persistedReducer, applyMiddleware(...middleware)); export const persistor = persistStore(store);
reducer :
const INIT_STATE = { hives: [], } const hiveReducer = (state = INIT_STATE, action) => { switch (action.type) { case SET_HIVES: return { ...state, hives: action.payload, }; [...]
action creator:
export const setHives = hives => { return { type: SET_HIVES, payload: hives, }; };
action:
export const getHives = () => { return dispatch => { axios.get(GET_HIVE_URL, HEADER).then(res => { const status = res.data.status; const hives = res.data.hives; if (status == 'hiveFound') { for (let i = 0; i < hives.length; i++) { console.log(hives[i]); dispatch(setHives(hives[i])); } } }); }; };
and my API send me:
"hives": [ { "hive_id": 123, "hive_name": "HelloHive" }, { "hive_id": 12944, "hive_name": null } ]
and console.log(hives[i]) return :
LOG {"hive_id": 123, "hive_name": "HelloHive"} LOG {"hive_id": 12944, "hive_name": null}
thanks you