How to save websocket frames in Chrome
Solution 1
Update for Chrome 63, January 2018
I managed to export them as JSON as this:
- detach an active inspector (if necessary)
- start an inspector on the inspector with ctrl-shift-j/cmd-opt-j
- paste the following code into that inspector instance.
At this point, you can do whatever you want with the frames. I used the console.save
utility from https://bgrins.github.io/devtools-snippets/#console-save to save the frames as a JSON file (included in the snippet below).
// https://bgrins.github.io/devtools-snippets/#console-save
(function(console){
console.save = function(data, filename){
if(!data) {
console.error('Console.save: No data')
return;
}
if(!filename) filename = 'console.json'
if(typeof data === "object"){
data = JSON.stringify(data, undefined, 4)
}
var blob = new Blob([data], {type: 'text/json'}),
e = document.createEvent('MouseEvents'),
a = document.createElement('a')
a.download = filename
a.href = window.URL.createObjectURL(blob)
a.dataset.downloadurl = ['text/json', a.download, a.href].join(':')
e.initMouseEvent('click', true, false, window, 0, 0, 0, 0, 0, false, false, false, false, 0, null)
a.dispatchEvent(e)
}
})(console)
// Frame/Socket message counter + filename
var iter = 0;
// This replaces the browser's `webSocketFrameReceived` code with the original code
// and adds two lines, one to save the socket message and one to increment the counter.
SDK.NetworkDispatcher.prototype.webSocketFrameReceived = function (requestId, time, response) {
var networkRequest = this._inflightRequestsById[requestId];
if (!networkRequest) return;
console.save(JSON.parse(response.payloadData), iter + ".json")
iter++;
networkRequest.addFrame(response, time, false);
networkRequest.responseReceivedTime = time;
this._updateNetworkRequest(networkRequest);
}
This will save all incoming socket frames to your default download location.
Solution 2
From Chrome 76 the HAR file now includes WebSocket messages.
WebSocket messages in HAR exports
The _webSocketMessages
property begins with an underscore to indicate that it's a custom field.
...
"_webSocketMessages": [
{
"type": "send",
"time": 1558730482.5071473,
"opcode": 1,
"data": "Hello, WebSockets!"
},
{
"type": "receive",
"time": 1558730482.5883863,
"opcode": 1,
"data": "Hello, WebSockets!"
}
]
...
Solution 3
This is something that is not possible to put into HAR format at this time because HAR specification does not have details on how to export framed transfer formats like WebSockets
From here: https://groups.google.com/forum/#!topic/google-chrome-developer-tools/jUOLFqpu-2Y
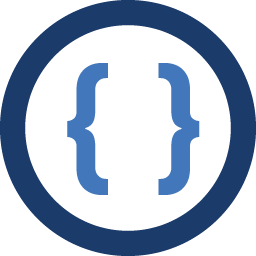
Admin
Updated on June 05, 2022Comments
-
Admin about 2 years
I am logging websocket traffic using Chrome/Developer Tools. I have no problem to view the websocket frames in network "Frames" window, but I can not save all frames (content enc. as JSON) in an external (text) file. I have already tried save as HAR and also simply used cntl A,C,V (first "page" copied only) but have so far not been very successful.
I am running Linux Mint 17.
Do you have hints how this can be done?
-
viksit over 6 yearsI get an SDK not defined when using your code. Any pointers on how to get that to work?
-
viksit over 6 yearsResolved this. For those who face this later - once you have the new inspector-inspector defined, you need to type in the code into the new window, but all your other WS activity is going to be output/recorded in the original console/browser window.
-
richardw almost 6 yearsIf you just want to dump all the frames from an existing wss frame log, you can do something like this:
console.save(BrowserSDK.networkLog.requests()[18]._frames, "frames.json")
-
bertrandg over 5 years@richardw hi, I want to do exactly what you said but can't, what's your chrome version? Can you tell more how you do please.
-
richardw over 5 years@bertrandg I'm now using
Version 71.0.3578.98 (Official Build) (64-bit)
and it looks likeBrowserSDK
is now justSDK
. I just tested it again with the following code:console.save(SDK.networkLog.requests()[27]._frames, "frames.json")
. Hope that helps. -
Cosimo over 5 years...and if you don't understand what "once you have the new inspector-inspector defined" means, do this: 1) Open a devtools window on the page performing the websockets traffic 2) Open a new tab to
chrome://inspect/#other
3) You should see a chrome-devtools item there now 4) Click "Inspect". A new devtools window will open. That is your inspector-inspector, that is, a devtools window that is inspecting the first devtools window. -
user202729 over 4 yearsSee also (for step 1): How do you inspect the web inspector in Chrome? - Stack Overflow