How to select a single contact from contacts in flutter
4,275
Solution 1
Add this dependenncy
This is the current version by this time
contact_picker: ^0.0.2
import 'package:flutter/material.dart';
import 'package:contact_picker/contact_picker.dart';
void main() {
runApp(new MyApp());
}
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => new _MyAppState();
}
class _MyAppState extends State<MyApp> {
final ContactPicker _contactPicker = new ContactPicker();
Contact _contact;
@override
Widget build(BuildContext context) {
return new MaterialApp(
home: new Scaffold(
appBar: new AppBar(
title: new Text('Plugin example app'),
),
body: new Center(
child: new Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
new MaterialButton(
color: Colors.blue,
child: new Text("CLICK ME"),
onPressed: () async {
Contact contact = await _contactPicker.selectContact();
setState(() {
_contact = contact;
});
},
),
new Text(
_contact == null ? 'No contact selected.' : _contact.toString(),
),
],
),
),
),
);
}
}
Solution 2
Use contact_picker: ^0.0.2
to get a contact
-
Add a dependency in your
pubspec.yaml
filedependencies: flutter: sdk: flutter contact_picker: ^0.0.2
-
Import it where you want to use it and create an openContactBook() function
import 'package:contact_picker/contact_picker.dart'; Future<String> openContactBook() async { Contact contact = await ContactPicker().selectContact(); if (contact != null) { var phoneNumber = contact.phoneNumber.number.toString().replaceAll(new RegExp(r"\s+"), ""); return phoneNumber; } return ""; }
-
Used it like this
onPressed: () { var contactNumber = openContactBook() }
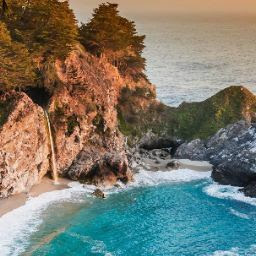
Author by
Shivam Srivastava
Updated on December 11, 2022Comments
-
Shivam Srivastava over 1 year
I have been stuck on this problem. How to select and fetch details from a contact in flutter? Please help.