how to select just one item from json in flutter
380
Solution 1
You can do this:
final lst = [{"ask":"28.47500","bid":"27.47500","name":"USDUAH","time":"1616772920.000"},
{"ask":"3972.06000","bid":"3971.16000","name":"SPX","time":"1617047940.000"},
{"ask":"0.71965","bid":"0.71916","name":"AUDCHF","time":"1617076421.403"}];
final res = lst.where((element) => element['name'] == 'SPX').toList();
Check out the full example on DartPad here
Solution 2
This might work
List data = parseResponse(response.body)
var spx ;
data.forEach((element) {
if (element["name"]=="SPX"){
spx =element; // access the spx object to get other data
}
});
static List<Trade> parseResponse(String responseBody) { // for converting array of objects to object list
final parsed = json.decode(responseBody).cast<Map<dynamic, dynamic>>();
return parsed.map<Trade>((json) => Trade.fromJson(json)).toList();
}```
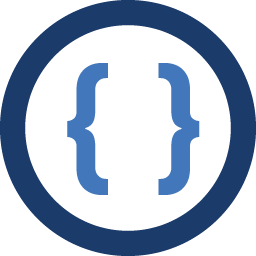
Author by
Admin
Updated on December 28, 2022Comments
-
Admin over 1 year
Hi i want to show SPX price in my app and have this json API i need to select just SPX data fron this API how can i do that??
[{"ask":"28.47500","bid":"27.47500","name":"USDUAH","time":"1616772920.000"}, {"ask":"3972.06000","bid":"3971.16000","name":"SPX","time":"1617047940.000"}, {"ask":"0.71965","bid":"0.71916","name":"AUDCHF","time":"1617076421.403"}]]