How to send a silent Push Notification payload
Solution 1
There are a few options for it! Let's take a small ride to understand all the different payloads and their usage.
Simple Payload
Displayed in Notification Center : Yes
Wakes app to perform background task : No
{
"aps" : {
"alert" : "You received simple notification!",
"badge" : 1,
"sound" : "default"
}
}
Payload With Custom Notification Sound
Displayed in Notification Center : Yes
Wakes app to perform background task : No
Step 1
: Add custom notification sound file (.wav or .aiff extensions only. e.g. notification.wav) in your app bundle.
Step 2
: Configure your payload as shown below to play your custom sound
{
"aps" : {
"alert" : "It's a custom notification sound!",
"badge" : 1,
"sound" : "notification.wav"
}
}
Notification With Custom Payload
Displayed in Notification Center : Yes
Wakes app to perform background task : No
{
"aps" : {
"alert" : "It's a notification with custom payload!",
"badge" : 1,
"content-available" : 0
},
"data" :{
"title" : "Game Request",
"body" : "Bob wants to play poker",
"action-loc-key" : "PLAY"
},
}
Here the data
dictionary holds custom information whatever you want. It will also display as normal notification with the alert message "It's a notification with custom payload!".
Normal Silent Notification
It will not a show an alert as a notification bar; it will only notify your app that there is some new data available, prompting the app to fetch new content.
Displayed in Notification center : No
Awake app to perform background task : Yes
{
"content-available" : 1
}
Silent Notification With Custom Payload
Here comes the magic to show a notification alert as well awake your app in background for a task! (Note: only if it's running in background and has not been killed explicitly by the user.)
Just add the extra parameter "content-available" : 1
in your payload.
Displayed in Notification Center : Yes
Wakes app to perform background task : Yes
{
"aps" : {
"alert" : "Notification with custom payload!",
"badge" : 1,
"content-available" : 1
},
"data" :{
"title" : "Game Request",
"body" : "Bob wants to play poker",
"action-loc-key" : "PLAY"
}
}
Use any of these payloads according to your app requirements. For background app refresh
refer to Apple's documentation. I hope this gives you all the necessary information. Happy coding :)
Solution 2
As i understand, you want extra data inside payload, so you can identify what push notification type is,or what action need to be handled.
For that edit your payload as:
$body = array(
'content-available' => 1,
'sound' => ''
);
$payload = array();
$payload['aps'] = $body;
$payload['action'] = 'order_update';
Then in your iOS Code:
- (void)application:(UIApplication *)application didReceiveRemoteNotification:(NSDictionary *)userInfo
{
NSString *action = userInfo["action"];
if([userInfo[@"aps"][@"content-available"] intValue]== 1 && [action isEqualToString:@"order_update") //order update notification
{
//handle Your Action here
return;
}
}
Hope this solves your problem!
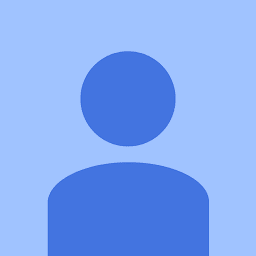
Sydney Loteria
Updated on April 14, 2021Comments
-
Sydney Loteria about 3 years
I just want to know how I can determine what action to do on a silent push:
This is the
aps
that I sent to the client:"aps": { "content-available": 1 }
My problem now is when I add
type: "Order_Update"
to determine that the silent push is for the Order Update to display an alert notification. -
Libor Zapletal over 7 yearsGreat answer but I think you miss data parameter in Notification with custom payload section.
-
Dipen Panchasara over 7 yearsThanks @LiborZapletal for noting it, i have updated answer.
-
makkasi over 7 yearsAccording the Apple documentation the "content-available' must be inside the "aps". developer.apple.com/library/content/documentation/… Why you use it outside of the "aps"?
-
Dipen Panchasara over 7 years@makkasi yes you are right, it should be under "aps", I have updated answer. Thanks for pointing out.
-
MarksCode about 7 yearsFor the Silent notification, can I have client-side logic to decide whether to display the notification or not?
-
Dipen Panchasara about 7 yearsNo you cant have client side logic as its decided by System based on payload , but based on payload "content-available" you can take some action
-
Alexander Larionov about 7 yearsI can't see any difference between 3rd example (Notification with custom payload) and 5th(Silent Notification with custom payload) example push dictionary. Why it causes different background execution behaviour, NO for the 3rd and YES for the 5th? They are both bring app to Background State and execute code, if some project settings enabled.
-
shadowmoses over 6 years@AlexanderLarionov I believe that the 3rd example should say "0" for content available, and "1" for the 5th example.That's the flag that adds the "silent" feature
-
Dipen Panchasara over 6 years@shadowmoses you are right, modified answer as suggested
-
fbenedet about 6 yearsI try to use the example "Silent notification with custom payload" but if the application is in foreground the system notification appear, i think if the alert is present it isn't silent notification. it is true?
-
Rachel Fong almost 6 years@DipenPanchasara thanks for the insight. When you say wakes app to perform background task, does the app trigger "applicationDidFinishLauchingWithOptions:" upon receiving the silent push?
-
Dipen Panchasara almost 6 years@rach No, it just invokes background task block which you have implemented for remote push-notification. It awakes app for particular task and suspends again, you can't perform long running task there. Hope it would be helpful :)
-
Teffi over 5 years@DipenPanchasara @rach It does call
applicationDidFinishLauchingWithOptions
. In our case the app is killed from the apps tray. But when a push notification withcontent-available 1
payload is received.applicationDidFinishLauchingWithOptions
is invoked. Another reference: stackoverflow.com/a/34728550/1045672 -
shaqir saiyed almost 3 yearsis sound parameter with 'defalut' is required to play ios custom sound ?