How to send an HTTPS GET Request in C#
164,514
Solution 1
Add ?var1=data1&var2=data2
to the end of url to submit values to the page via GET:
using System.Net;
using System.IO;
string url = "https://www.example.com/scriptname.php?var1=hello";
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(url);
HttpWebResponse response = (HttpWebResponse)request.GetResponse();
Stream resStream = response.GetResponseStream();
Solution 2
I prefer to use WebClient, it seems to handle SSL transparently:
http://msdn.microsoft.com/en-us/library/system.net.webclient.aspx
Some troubleshooting help here:
https://clipperhouse.com/webclient-fiddler-and-ssl/
Solution 3
Simple Get Request using HttpClient Class
using System.Net.Http;
class Program
{
static void Main(string[] args)
{
HttpClient httpClient = new HttpClient();
var result = httpClient.GetAsync("https://www.google.com").Result;
}
}
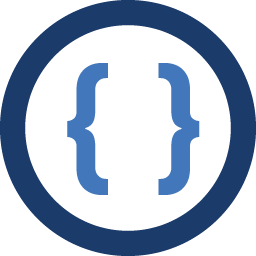
Author by
Admin
Updated on February 02, 2020Comments
-
Admin over 4 years
Related: how-do-i-use-webrequest-to-access-an-ssl-encrypted-site-using-https
How to send an HTTPS GET Request in C#?
-
Pavdro over 9 yearsNote: this works for HTTP, I tested this code with HTTPS url and it didn't work. Going to try WebClient and see if that works with SSL
-
Bitterblue almost 6 yearsUse the address of this page. Stackoverflow runs on https and you will get a
200 OK
responsestring url = "https://stackoverflow.com/questions/...";
. Worked! -
Yituo over 5 yearsWill this be a http call or an https call?
-
taimoor1990 over 3 yearsThis will result in an exception saying SLS/TLS issue.
-
Alexander Ryan Baggett about 3 yearsPost actual code, links can become stale.
-
Michiel almost 3 yearsNote that WebRequest and HttpWebRequest are no longer recommended to use for new projects. Microsoft suggests using the newer HttpClient class instead: docs.microsoft.com/en-us/dotnet/api/system.net.http.httpclient