How to send an image as a blob from Angular?
11,539
Solution 1
You can upload the file like this:
TS
import { HttpClient } from "@angular/common/http";
import { Injectable } from "@angular/core";
import { Component, OnInit } from '@angular/core';
@Injectable({
providedIn: "root"
})
export class UploadService {
constructor(private httpClient: HttpClient) {
}
public uploadFile<T>(file: File): Observable<T> {
let formData = new FormData();
formData.append('file', file, file.name);
var request = this.httpClient
.post<T>(
"url/to/backend/upload",
formData
);
return request;
}
}
@Component({
selector: 'app-carga',
templateUrl: './carga.component.html',
template:
`
<input class="form-control" #fileInput type="file" [multiple]="false"/>
<button type="button" class="btn btn-outline-success"
(click)="uploadFile(fileInput)">Cargar</button>
`
})
export class CargaComponent implements OnInit {
constructor(public uploader: UploadService) {
}
ngOnInit(): void {
}
public uploadResult?: any;
async uploadFile(fileInput: any) {
let files: File[] = fileInput.files;
if (files.length < 1) {
return;
}
let file = files[0];
try {
this.uploader.uploadFile(file)
.subscribe(result => {
console.log(result);
fileInput.value = null;
},
error => {
console.error(error);
})
} catch (error) {
console.warn("File upload failed.");
console.error(error);
}
}
}
Solution 2
I agree with comment above . This is an example for you
Or you can use dataForm as below:
uploadPicture(formData: FormData, code: string) {
// /** In Angular 5, including the header Content-Type can invalidate your request */
const headers = new HttpHeaders();
headers.append('Content-Type', null);
headers.append('Accept', 'application/json');
const options = {
headers: headers
};
const url = this.xxxServiceURL + '/custom/xxx/uploadPicture/' + code;
return this.httpClient.post(url, formData, options);
}
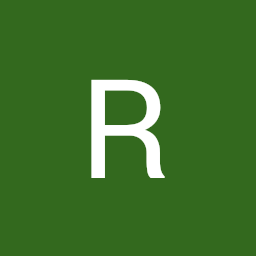
Author by
joler-botol
Updated on June 26, 2022Comments
-
joler-botol almost 2 years
I am using a file input tag to get the image file. Now, I want to send it as a blob which I want to store in the database as a String. I will retrieve it and render it as an image with a get call.
But how do I post the image as a blob?