How to send argument to class in Quartz.Net
Solution 1
You can use JobDataMap
jobDetail.JobDataMap["jobSays"] = "Hello World!";
jobDetail.JobDataMap["myFloatValue"] = 3.141f;
jobDetail.JobDataMap["myStateData"] = new ArrayList();
public class DumbJob : IJob
{
public void Execute(JobExecutionContext context)
{
string instName = context.JobDetail.Name;
string instGroup = context.JobDetail.Group;
JobDataMap dataMap = context.JobDetail.JobDataMap;
string jobSays = dataMap.GetString("jobSays");
float myFloatValue = dataMap.GetFloat("myFloatValue");
ArrayList state = (ArrayList) dataMap["myStateData"];
state.Add(DateTime.UtcNow);
Console.WriteLine("Instance {0} of DumbJob says: {1}", instName, jobSays);
}
}
Solution 2
To expand on @ArsenMkrt's answer, if you're doing the 2.x-style fluent job config, you could load up the JobDataMap
like this:
var job = JobBuilder.Create<MyJob>()
.WithIdentity("job name")
.UsingJobData("x", x)
.UsingJobData("y", y)
.Build();
Solution 3
Abstract
Let me to extend a bit @arsen-mkrtchyan post with significant note which might avoid a painful support Quartz code in production:
Problem (for persistance JobStore)
Please remember about JobDataMap versioning in case you're using persistent JobStore, e.g. AdoJobStore.
Summary (TL;DR)
- Carefully think on constructing/editing your JobData otherwise it will lead to issues on triggering future jobs.
- Enable “quartz.jobStore.useProperties” config parameter as official documentation recommends to minimize versioning problems. Use JobDataMap.PutAsString() later.
Details
It's also stated in the documentation, however, not so highlighted, but might lead to big maintenance problem if e.g. you removing some parameter in the next version of you app:
If you use a persistent JobStore (discussed in the JobStore section of this tutorial) you should use some care in deciding what you place in the JobDataMap, because the object in it will be serialized, and they therefore become prone to class-versioning problems.
Also there is related note about configuring JobStore mentioned in the relevant document:
The “quartz.jobStore.useProperties” config parameter can be set to “true” (defaults to false) in order to instruct AdoJobStore that all values in JobDataMaps will be strings, and therefore can be stored as name-value pairs, rather than storing more complex objects in their serialized form in the BLOB column. This is much safer in the long term, as you avoid the class versioning issues that there are with serializing your non-String classes into a BLOB.
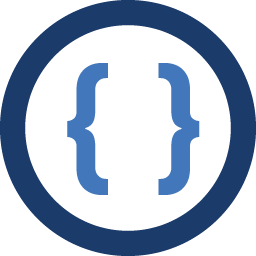
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I'm using Quartz.Net (version 2) for running a method in a class every day at 8:00 and 20:00 (IntervalInHours = 12)
Everything is OK since I used the same job and triggers as the tutorials on Quartz.Net, but I need to pass some arguments in the class and run the method bases on those arguments.
Can any one help me how I can use arguments while using Quartz.Net?