How to send raw text with xmlhttp request?
Solution 1
Yes it can be done by modifying headerRequest
and data
like this:
var xhr = (window.XMLHttpRequest) ? new XMLHttpRequest() : new activeXObject("Microsoft.XMLHTTP");
if(typeof(FormData) == 'undefined'){
var boundary = '---------------------------' + (new Date).getTime(),//boundary is used to specify the encapsulation boundary of a parameter
data = "--" + boundary + "\r\n";
data += 'Content-Disposition: form-data; name="data"\r\n\r\n';//here we specify the name of the parameter name (data) sent to the server which can be retrieved by $_POST['data']
data += string + "\r\n";
data += "--" + boundary + "--\r\n";
xhr.open( 'post', 'writeCode.php', true );
xhr.setRequestHeader('Content-Type', 'multipart/form-data; boundary=' + boundary);
}else{
var data = new FormData();
data.append("data", string);
xhr.open( 'post', 'writeCode.php', true );
}
xhr.send(data);
Solution 2
If using FormData
works correctly for you, then in situations where you can't use it, actually all you need to do is to replace your last line with:
xhr.send("data="+encodeURIComponent(string));
I think the other answerers were confused by your asking that the text "not be encoded". Form data is generally encoded for sending over HTTP, but then decoded by PHP when it arrives at the server, entirely transparently: you get exactly the original text back, no matter what special characters it might contain. (Assuming you interpret the PHP string as UTF-8).
So, following your example, if your PHP file contained:
$data = $_POST['data'];
Then the contents of the PHP $data
variable would be the string '<td clas="tdClass">some text<?php echo $contents; ?></td>'
. This is the same as if you'd used the FormData
method.
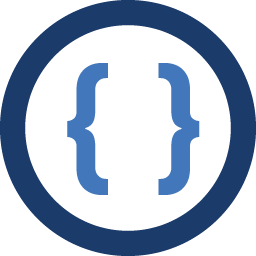
Admin
Updated on July 20, 2022Comments
-
Admin almost 2 years
I'm trying to send a
textarea
field's text to a PHP file using ajax, the text contains HTML characters and should not be encoded.
UsingFormData
it works perfectly however it's not supported in ie 9 and older versions! I tried to send the data asstring
by setting therequestHeader
totext/plain;charset=UTF-8;
ormultipart/form-data
but it didn't work! the code i'm using is:var string = '<td clas="tdClass">some text<?php echo $contents; ?></td>'; var data = new FormData(); data.append("data" , string); var xhr = (window.XMLHttpRequest) ? new XMLHttpRequest() : new activeXObject("Microsoft.XMLHTTP"); xhr.open( 'post', '/path/to/php', true ); xhr.send(data);
what's an alternative way to do this in IE 9?