How to serialize object to CSV file?
Solution 1
First, serialization is writing the object to a file 'as it is'. AFAIK, you cannot choose file formats and all. The serialized object (in a file) has its own 'file format'
If you want to write the contents of an object (or a list of objects) to a CSV file, you can do it yourself, it should not be complex.
Looks like Java CSV Library can do this, but I have not tried this myself.
EDIT: See following sample. This is by no way foolproof, but you can build on this.
//European countries use ";" as
//CSV separator because "," is their digit separator
private static final String CSV_SEPARATOR = ",";
private static void writeToCSV(ArrayList<Product> productList)
{
try
{
BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(new FileOutputStream("products.csv"), "UTF-8"));
for (Product product : productList)
{
StringBuffer oneLine = new StringBuffer();
oneLine.append(product.getId() <=0 ? "" : product.getId());
oneLine.append(CSV_SEPARATOR);
oneLine.append(product.getName().trim().length() == 0? "" : product.getName());
oneLine.append(CSV_SEPARATOR);
oneLine.append(product.getCostPrice() < 0 ? "" : product.getCostPrice());
oneLine.append(CSV_SEPARATOR);
oneLine.append(product.isVatApplicable() ? "Yes" : "No");
bw.write(oneLine.toString());
bw.newLine();
}
bw.flush();
bw.close();
}
catch (UnsupportedEncodingException e) {}
catch (FileNotFoundException e){}
catch (IOException e){}
}
This is product (getters and setters hidden for readability):
class Product
{
private long id;
private String name;
private double costPrice;
private boolean vatApplicable;
}
And this is how I tested:
public static void main(String[] args)
{
ArrayList<Product> productList = new ArrayList<Product>();
productList.add(new Product(1, "Pen", 2.00, false));
productList.add(new Product(2, "TV", 300, true));
productList.add(new Product(3, "iPhone", 500, true));
writeToCSV(productList);
}
Hope this helps.
Cheers.
Solution 2
For easy CSV access, there is a library called OpenCSV. It really ease access to CSV file content.
EDIT
According to your update, I consider all previous replies as incorrect (due to their low-levelness). You can then go a completely diffferent way, the hibernate way, in fact !
By using the CsvJdbc driver, you can load your CSV files as JDBC data source, and then directly map your beans to this datasource.
I would have talked to you about CSVObjects, but as the site seems broken, I fear the lib is unavailable nowadays.
Solution 3
It would be interesting to have a csv serializer as it would take up the minimal space compared to other serializing method.
The closest support for java object to csv is stringutils provided by spring utils project
arrayToCommaDelimitedString(Object[] arr) but it is far from being a serializer.
Here is a simple utility which uses reflection to serialize value objects
public class CSVWriter
{
private static String produceCsvData(Object[] data) throws IllegalArgumentException, IllegalAccessException, InvocationTargetException
{
if(data.length==0)
{
return "";
}
Class classType = data[0].getClass();
StringBuilder builder = new StringBuilder();
Method[] methods = classType.getDeclaredMethods();
for(Method m : methods)
{
if(m.getParameterTypes().length==0)
{
if(m.getName().startsWith("get"))
{
builder.append(m.getName().substring(3)).append(',');
}
else if(m.getName().startsWith("is"))
{
builder.append(m.getName().substring(2)).append(',');
}
}
}
builder.deleteCharAt(builder.length()-1);
builder.append('\n');
for(Object d : data)
{
for(Method m : methods)
{
if(m.getParameterTypes().length==0)
{
if(m.getName().startsWith("get") || m.getName().startsWith("is"))
{
System.out.println(m.invoke(d).toString());
builder.append(m.invoke(d).toString()).append(',');
}
}
}
builder.append('\n');
}
builder.deleteCharAt(builder.length()-1);
return builder.toString();
}
public static boolean generateCSV(File csvFileName,Object[] data)
{
FileWriter fw = null;
try
{
fw = new FileWriter(csvFileName);
if(!csvFileName.exists())
csvFileName.createNewFile();
fw.write(produceCsvData(data));
fw.flush();
}
catch(Exception e)
{
System.out.println("Error while generating csv from data. Error message : " + e.getMessage());
e.printStackTrace();
return false;
}
finally
{
if(fw!=null)
{
try
{
fw.close();
}
catch(Exception e)
{
}
fw=null;
}
}
return true;
}
}
Here is an example value object
public class Product {
private String name;
private double price;
private int identifier;
private boolean isVatApplicable;
public Product(String name, double price, int identifier,
boolean isVatApplicable) {
super();
this.name = name;
this.price = price;
this.identifier = identifier;
this.isVatApplicable = isVatApplicable;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(long price) {
this.price = price;
}
public int getIdentifier() {
return identifier;
}
public void setIdentifier(int identifier) {
this.identifier = identifier;
}
public boolean isVatApplicable() {
return isVatApplicable;
}
public void setVatApplicable(boolean isVatApplicable) {
this.isVatApplicable = isVatApplicable;
}
}
and the code to run the util
public class TestCSV
{
public static void main(String... a)
{
Product[] list = new Product[5];
list[0] = new Product("dvd", 24.99, 967, true);
list[1] = new Product("pen", 4.99, 162, false);
list[2] = new Product("ipad", 624.99, 234, true);
list[3] = new Product("crayons", 4.99,127, false);
list[4] = new Product("laptop", 1444.99, 997, true);
CSVWriter.generateCSV(new File("C:\\products.csv"),list);
}
}
Output:
Name VatApplicable Price Identifier
dvd true 24.99 967
pen false 4.99 162
ipad true 624.99 234
crayons false 4.99 127
laptop true 1444.99 997
Solution 4
Two options I just ran into:
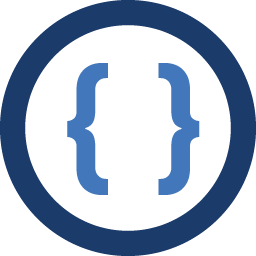
Admin
Updated on July 11, 2020Comments
-
Admin almost 4 years
I want to write a Object into CSV file. For XML we have XStream like this
So if i want to convert object to CSV do we have any such library ?EDIT: I want to pass my list of Bean to a method which should write all the fields of bean to CSV.