How to set a global variable in python flask?
Solution 1
You don't need a global variable for this and truly speaking you won't need them anytime in the future as it is a bad practice to use global variables. Refer to this link for details, why are global variables evil?
Now coming to your problem, you probably need g
module of flask which creates a context which persist over multiple requests from the same user. You can do something like this:
from flask import g
...
def get_messages():
messages = getattr(g, '_messages', None)
if messages is None:
g._messages = [] # to store messages you may use a dictionary
return g._messages
def add_message(message):
messages = get_messages()
messages.append(message)
setattr(g, '_messages', messages)
return messages
And remember for each user a different thread of the app is created so the neither the variables are shared nor their values. So for each user there will be a different g
but it will persist over multiple requests from the same user. Hope it helps!
Edit: g
object is a proxy to application context and doesn't persists across multiple requests. It persists for a single requests across the application.
Solution 2
Not sure if I'm understanding your question correctly, but perhaps you can create a global dictionary with key as the username and value as the message.
So each time you want to update the variable in the dictionary you can access the respective variable based on the username (key of the dictionary)
userMsg = {"John":"hello"} #existing user
# update John's msg
userMsg["John"] = "bye"
# now userMsg is {"John":"bye"}
# add new user if user does not exist
userMsg["Mary"] = "Your Message Here"
# now userMsg is {"John":"bye", "Mary":"Your Message Here"}
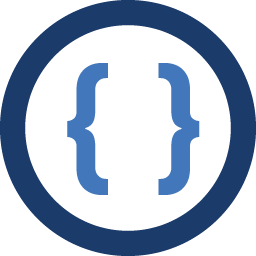
Admin
Updated on June 14, 2022Comments
-
Admin almost 2 years
I would like to set a global variable and use it as a trigger of various functions. Each user has a separate global variable. This is used to keep track of previous message data and proceed a conversation. The problem is that how can I manage a separate global variable to each user? The app is running once I deployed it in the server. When I attempt to change the global variable, this variable applies to every user, not just that single user who triggered its the change.
I'm using python flask without a DB.
Thank you.