How to set a specific starting volume for <audio> tag
Solution 1
You're not getting the element, and the error shows up in the console
Cannot set property 'volume' of null
Move the script to right before the </body>
tag, or do :
window.onload = function() {
var backgroundAudio=document.getElementById("bgAudio");
backgroundAudio.volume=0.5;
}
EDIT:
Nothing is more annoying than disabling right clicks, but anyway, here's what you have
;(function($){
mySong=document.getElementById("bgAudio");
mySong.volume=0.2;
setVolume = function(bgAudio,vol) {
sounds[bgAudio].volume = 0.33;
}
})(jQuery);
now change it to
jQuery(function($) {
$("#bgAudio").prop("volume", 0.2);
window.setVolume = function(bgAudio, vol) {
sounds[bgAudio].volume = vol;
}
});
Solution 2
Use JavaScript to set the volume, however you need to make sure to have the DOM ready to modify any objects. Perhaps use an ondocumentready event, or a Click event from a call-to-action.
First, get the video object you want to modify.
bgAudio = document.getElementById("bgAudio");
Then use the bgAudio object to set its properties, like the volume.
bgAudio.volume = 0.2;
Since you are working on a website, I would do something like this (assuming you can use jQuery):
$(document).ready(function() {
// the site has now loaded, grab the video!
bgAudio = document.getElementById("bgAudio");
// now tweak the volume!
bgAudio.volume = 0.2;
// now, play it!
bgAudio.play();
});
Check out this reference material on jquery ready, events and videos:
- http://api.jquery.com/ready/
- https://developer.mozilla.org/en-US/docs/Web/Reference/Events
- https://developer.mozilla.org/en-US/docs/Web/HTML/Element/video
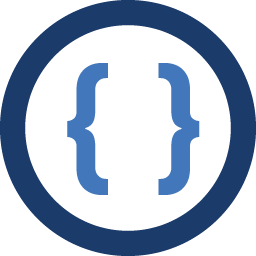
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I only have basic knowledge of HTML and CSS and have looked through Google extensively trying to find an answer to this question -- it all seems to point to JavaScript and/or jQuery. I tried it, and I can't get it to work.
I have an audio file that starts to play when my website loads. I want to set a specific starting volume so that my visitors don't get a heart attack if their volume is high.
This is what I have:
HTML:
<audio id="bgAudio" autoplay="autoplay" controls="controls" loop="loop" src="audio/025.mp3"></audio>
CSS:
#bgAudio { position:fixed; z-index:1000; width:250px; }
JavaScript:
backgroundAudio=document.getElementById("bgAudio"); backgroundAudio.volume=0.5;
Any help would be greatly appreciated!