How to set an "empty" element in any part of an Array in C
Solution 1
This is simply not possible in C. One workaround might be to change the array elements into a struct having a field used
being a boolean and a field data
being an int.
Solution 2
You can use another sentinel value to represent "not used". Say INT_MAX
or INT_MIN
.
Solution 3
You can use negative values for instance. But it's really dirty hack).
Solution 4
NULL is a pointer. Assigning it or comparing to any other type (like int
) should at least produce a warning from the compiler. Do you enter pointers from the console?
Integer scalars mostly do not have reserved codes (and if, that would be implementation defined). So you need something out-of-band to signal "empty".
YOu can either restrict the valid range of values and use a value outside that range as "empty" tag. Or use an additional flag per entry. For float, there are similar ways, e.g. using NaN.
Version with seperate flag using a struct:
#include <stdbool.h>
struct {
bool valid;
int value;
} array[20];
Version with INT_MIN as reserved value:
#include <limits.h>
#define VALID_MIN (INT_MIN - 1)
#define EMPTY_VALUE INT_MIN
for ( int i = 0 ; i < sizeof(array) /sizeof(array[0]) ; i++ )
array[i] = EMPTY_VALUE;
....
int value;
do {
value = input_value(); // ...
} while ( value < VALID_MIN ) ;
....
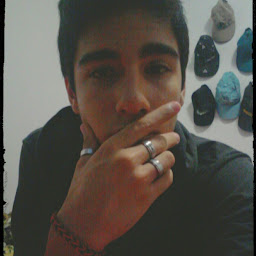
Skepller
Updated on June 05, 2022Comments
-
Skepller almost 2 years
I'm doing a program to insert numbers in an array, simple thing here, for example:
if(menu==1){ system("cls"); result=lastposition(array,20); if(result==25){ printf("\n Error! The array is FULL!"); printf("\n Press Enter to continue"); getch(); } else{ printf("\n\n\tOption 1 selected: \n"); printf("\n Type the number to add it to the array: "); scanf("%i",&value); if(array[0]!=NULL){ printf("\n The first space is already filled, moving itens"); changeplace(array,20,value); printf("\n Items moved with success!\n"); }else { array[0] = value; } printf("\n Number %i added!\n",value); printf(" Press to continue.\n"); getch(); }
So, what this does is, you type a number, and it inserts in a 20 positions array, in its first position (that's why the function "lastposition" is there, to check the lastposition filled in the array), if the first position is not filled yet(NULL), it adds in the first position, if it is already filled, the "chageplace" function moves all the values to the right, and then adds the number!
My problem is, when i declared the array, i set all its values to NULL, like this:
int array[20]={NULL};
! But in C, NULL change all its values to 0, and if someone in my program add 0 as a value, lets say the array already got a 5 and a 10 on it, like this[5][10][0][0][0]...[0]
, I'll add the zero, getting like this:[0][5][10][0][0]...[0]
, till there ok, but because C considers NULL=0, when i try to add another number it will check if the first position is already filled (it'll check if it's NULL, or 0, and it will return as NULL [0]), and if i try to add a 7 on it, it will replace the 0 (the added number, not the NULL) and it will get like[7][5][10][0][0]...[0]
Anyone knows how to fix this? Someway to be able to add a 0 in the function, without it being replaced by the next number because it's considered NULL? Having a real "Nothing" there, instead of "0".
PS: I cant just put random numbers in the array, to be different of 0, for 2 reasons, first, option 3 of the menu show the vector [therefore showing the random number, and 2, my function would consider it as already filled by a number!
PS²: The "25" that the "lastposition" function returns is just a random number I've set to return if the last position of the array is 20 (meaning its already full)...