How to set cookies in laravel 5 independently inside controller
Solution 1
You may try this:
Cookie::queue($name, $value, $minutes);
This will queue the cookie to use it later and later it will be added with the response when response is ready to be sent. You may check the documentation on Laravel
website.
Update (Retrieving A Cookie Value
):
$value = Cookie::get('name');
Note: If you set a cookie in the current request then you'll be able to retrieve it on the next subsequent request.
Solution 2
If you want to set cookie and get it outside of request, Laravel is not your friend.
Laravel cookies are part of Request, so if you want to do this outside of Request object, use good 'ole PHP setcookie(..) and $_COOKIE to get it.
Solution 3
You are going right way my friend.Now if you want retrive cookie
anywhere in project just put this code $val = Cookie::get('COOKIE_NAME');
That's it!
For more information how can this done click here
Solution 4
Here is a sample code with explanation.
//Create a response instance
$response = new Illuminate\Http\Response('Hello World');
//Call the withCookie() method with the response method
$response->withCookie(cookie('name', 'value', $minutes));
//return the response
return $response;
Cookie can be set forever by using the forever method as shown in the below code.
$response->withCookie(cookie()->forever('name', 'value'));
Retrieving a Cookie
//’name’ is the name of the cookie to retrieve the value of
$value = $request->cookie('name');
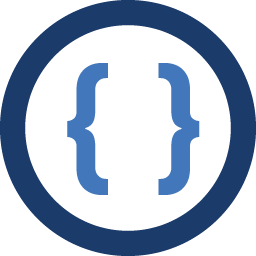
Admin
Updated on May 09, 2020Comments
-
Admin almost 4 years
I want to set cookies in Laravel 5 independently
i.e., Don't want to use
return response($content)->withCookie(cookie('name', 'value'));
I just want to set cookie in some page and retrieve in some other page
Creation can be like this
$cookie = Cookie::make('name', 'value', 60);
But how can i retrieve those cookies in some controller itself ?
-
The Alpha almost 9 yearsPlease check the documentation (link given in the answer), you'll find everything there.
-
Dan Jones over 7 yearsHe asked for Laravel 5. This example is for Laravel4.
-
Dan Jones over 7 yearsDoesn't work on my Laravel 5. That
Cookie
class seems to be gone. -
The Alpha over 7 years@DanJones, I'll check soon, which release (5.0/5.2/5.2...) of version - 5 you are on?
-
Dan Jones over 7 years5.0.35 Although, I'm still pretty new to Laravel, so there may be something I'm missing.
-
Nil over 7 yearsHi, I use $value=Cookie::get('name'); but when I echo $value it shows me blank, before this I set cookie by using Cookie::queue('name',$name); Plz help me to resolve this
-
Jigs Virani over 7 years$value will be null if cookie not present. see this link: laravel-recipes.com/recipes/49/…
-
Jeffz over 6 years@Jigs Virani You are wrong. If you want to set cookie and get it in Laravel outside of request, Laravel is not your friend. Even link you gave says; "This is basically the same as Request::cookie()". So, Nil is right to complain.
-
Kokodoko over 4 yearsI'm getting "maximum execution time exceeded" when using this.
-
t q over 3 years
setcookie()
is not working, are there any settings that may be interfering this?