How to set/modify model in controller
Solution 1
Go for setupController
hook in your route
App.IndexRoute = Em.Route.extend({
model: function() {
return Em.$.get('data.json');
},
setupController: function(controller, model) {
this._super(controller, model);
//Some modifications
//..
//end of modificaitons
controller.set('model', model);
}
});
Solution 2
extend()
is used to create subclass for any class, for instance, Ember.ArrayController
. If you want to overwrite the properties (methods) of the existing class you can go with extend.
create()
is used to create new instance of a class. Refer this http://emberjs.com/guides/object-model/classes-and-instances/
No need to use setupController
hook of Route
until you want to set any properties for the controller.
If your Route have model hook and return data, then you can access model directly in your controller by using
var model = this.get('model');
At the same time you can use setters to update/modify model.
this.set('model',newdata);
Have a look at simple bin http://jsbin.com/fesux/edit
Kindly have a look at Ember.set and Ember.get also
Solution 3
You can utilize a setupController to handle this
App.IndexRoute=Em.Route.extend({
model:function(){
return Em.$.get('data.json');
},
setupController: function(controller, model){
controller.set('model',model);
}
}
The setupController hook receives the route handler's associated controller as its first argument. In this case, the IndexRoute's setupController receives the application's instance of App.IndexController.
The default setupController hook sets the model property of the associated controller to the route handler's model.
If you want to configure a controller other than the controller associated with the route handler, use the controllerFor method.
More details here: http://emberjs.com/guides/routing/setting-up-a-controller/
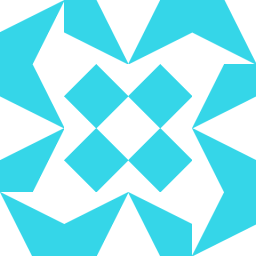
Comments
-
rain 6 months
I'm doing a basic example for ajax operation in ember 1.7.0, What I did is have a model in route and did a ajax get for raw data.
App.IndexRoute=Em.Route.extend({ model:function(){ return Em.$.get('data.json'); }, }
Now in controller I want to modify this for the template, I tried
App.IndexController = Ember.Controller.extend({ init:function(){this._super(); var newmodel=this.get('content'); ....some modification... this.set('model',newmodel); } }
but this is not working.
So how basically One modify the model? This should be in setupController or in controller? if need be how to get and set model in controller? Another confusion is on when to create and when to extend the controller and route?
Thanks.