How to set Toolbar background color in an activity to a color inside colors.xml file?
22,474
Solution 1
Use this code
getSupportActionBar().setBackground(new ColorDrawable(getResources().getColor(R.color.white)));
Solution 2
Here is code for Kotlin
supportActionBar!!.setBackgroundDrawable(ColorDrawable(resources.getColor(R.color.colorPrimary)))
Solution 3
Try creating new layout resource toolbar.xml:
<?xml version="1.0" encoding="utf-8"?>
<android.support.v7.widget.Toolbar
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@color/MAIN_A" />
And then include it in your activity layout like this:
<include
android:id="@+id/toolbar"
layout="@layout/toolbar" />
Then you need to set this toolbar in your activity onCreate method:
toolbar = (Toolbar) findViewById(R.id.toolbar);
if (toolbar != null) {
// set toolbar object as actionbar
setSupportActionBar(toolbar);
}
After that you can access your new action bar from getSupportActionBar() method. Tell me if it helps :)
Solution 4
First, ActionBar is depricated, use Toolbar (android.widget.Toolbar) instead. If this is not possible, try the support of ActionBar as follows :
android.support.v7.app.ActionBar actionBar = getSupportActionBar();
actionBar.setBackgroundDrawable(new ColorDrawable(getResources().getColor(R.color.MAIN_A)));
For the toolbar, it's :
toolbar.setBackgroundResource(R.color.MAIN_A)
Solution 5
Try it like that:
ActionBar actionBar = getActionBar();
actionBar.setBackgroundDrawable(new ColorDrawable(getResources().getColor(R.color.MAIN_A)));
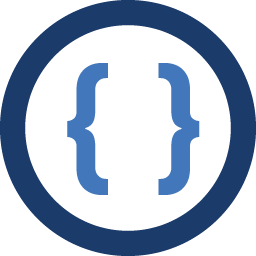
Author by
Admin
Updated on July 31, 2022Comments
-
Admin almost 2 years
I have a color in my
colors.xml
file which I need to use fortoolbar
color<resources> <color name="MAIN_A">#f16264</color> </resources>
Now I need to use
MAIN_A
as a color fortoolbar
. -
Admin over 8 yearshow do I change the color bacground for Toolbar??
-
Hichem Acher over 8 years@user5148540 toolbar.setBackgroundResource(R.color.MAIN_A)
-
CoolMind about 6 yearssetBackgroundDrawable is deprecated.
-
GabrielBB over 5 years@CoolMind Not true. It is deprecated in other classes but not in ActionBar.class
-
CoolMind over 5 years@GabrielBB, hmm, maybe you are right, but
ActionBar
itself is deprecated and heregetSupportActionBar()
is used.