How to set up Xcode to run OpenCL code, and how to verify the kernels before building
Solution 1
Long answer for command liners:
With a recent Xcode (and Lion or higher), you don't even need to call clCreateProgramWithSource
, just write your kernel and call it from your app, there are some additional compiling steps needed though.
I'm taking the OpenCL Hello World example from Apple here using default compiler flags (see https://developer.apple.com/library/mac/#documentation/Performance/Conceptual/OpenCL_MacProgGuide/ExampleHelloWorld/Example_HelloWorld.html) and showing the steps Xcode would do in the background.
To get you started, do a:
/System/Library/Frameworks/OpenCL.framework/Libraries/openclc -x cl -cl-std=CL1.1 -cl-auto-vectorize-enable -emit-gcl mykernel.cl
This will create 2 files, mykernel.cl.h and mykernel.cl.c (and mykernel.cl.c does all the magic of loading your kernel into the app). Next you'll need to compile the kernel:
/System/Library/Frameworks/OpenCL.framework/Libraries/openclc -x cl -cl-std=CL1.1 -Os -triple i386-applecl-darwin -emit-llvm-bc -o mykernel.cl.i386.bc mykernel.cl
/System/Library/Frameworks/OpenCL.framework/Libraries/openclc -x cl -cl-std=CL1.1 -Os -triple x86_64-applecl-darwin -emit-llvm-bc -o mykernel.cl.x86_64.bc mykernel.cl
/System/Library/Frameworks/OpenCL.framework/Libraries/openclc -x cl -cl-std=CL1.1 -Os -triple gpu_32-applecl-darwin -emit-llvm-bc -o mykernel.cl.gpu_32.bc mykernel.cl
And last but not least, build the app itself:
clang -c -Os -Wall -arch x86_64 -o mykernel.cl.o mykernel.cl.c
clang -c -Os -Wall -arch x86_64 -o square.o square.c
clang -framework OpenCL -o square mykernel.cl.o square.o
And that's it. You won't see any clCreateProgramWithBinary
or clCreateProgramWithSource
in Apple's sample code. Everything is done via mykernel.cl.c generated by openclc
.
Solution 2
To answer the second part of your question, on Mountain Lion you can run the OpenCL compiler on foo.cl
like this:
/System/Library/Frameworks/OpenCL.framework/Libraries/openclc \
-c -Wall -emit-llvm -arch gpu_32 -o foo.bc foo.cl
It will compile foo.cl
to LLVM bit-code foo.bc
. This binary file can be used with clCreateProgramWithBinary
, which is faster than clCreateProgramWithSource
. Valid values for -arch
are i386 x86_64 gpu_32
.
Related videos on Youtube
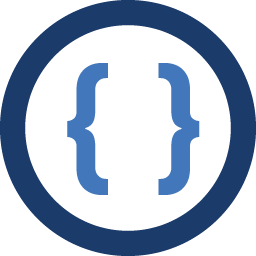
Admin
Updated on October 06, 2022Comments
-
Admin over 1 year
I am looking at the official documentation on the Apple site, and I see that there is a quickstart about how to use OpenCL on Xcode.
Maybe it is just me, but I had no luck building the code that is mentioned on the "hello world OCL" section.
I've started Xcode and created an empty project; created a main.c and a .cl kernel file, pasting what is on the Apple developer site, and I am not able to get anything to build, even after adding a target.
The AD site does not have a project to download, so I have no clue about the cause of the failure (it may be me most likely, or the site assume steps and does not mention them).
I've also tried the sample project from macresearch.org, but they are quite ancient, and the test project in the 3rd lesson is not running at all.
Now, I am pretty sure that others are using Xcode to run OCL code, but I cannot find any single page (except the aforementioned macresearch.org) that gives a clear setup about how to run an Xcode project with OCL. Is there anyone aware of a tutorial that shows how to work with OCL and Xcode?
I have purchased 3 books on OCL (Gaster's Heterogeneous computing with OpenCL, Scarpino's OpenCL in action and Munshi's OpenCL programming guide), and neither mention how to set up Xcode, while they go in detail for the visual studio setup and even for the eclipse setup.
On the side; is there any application that is able to validate kernel code before running it in the OCL application?
Thanks in advance for any suggestion that you may have.
-
Admin over 11 yearsThanks a lot Eric! This will be useful!
-
Amir about 7 yearsThanks for this answer. Could you let me know what is the different between having gcc/llvm compile the opencl code using its opencl library versus the method you described?