How To Shuffle String Arrays
13,417
Solution 1
If you task isn’t implementation of the snuffle algorithm you can use standard java.util.Collections#shuffle method:
String[] firstName = new String[] {"a","b","c","d","e"};
List<String> strList = Arrays.asList(firstName);
Collections.shuffle(strList);
firstName = strList.toArray(new String[strList.size()]);
Solution 2
just create an array (or list) which has the values
Integer[] arr = new Integer[firstName.length];
for (int i = 0; i < arr.length; i++) {
arr[i] = i;
}
Collections.shuffle(Arrays.asList(arr));
and then just create a new string array to move the values into
string[] newFirstName = new string[arr.length]();
for(int i=0; i < arr.length; i++)
{
newFirstName[i] = firstName[arr[i]];
//etc ....
}
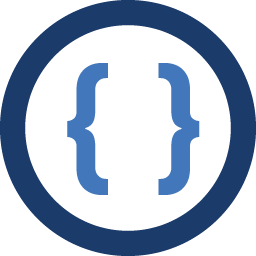
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
How Can I Shuffle below array
String[] firstName =["a","b","c","d","e"]; String[] lastName =["p","q","r","s","t"]; String[] salary =["10","20","30","40",50"]; String[] phoneNo= ["1","2","3","4","5"];
after shuffling the array i need result like
String[] firstName =["d","b","e","c","a"]; String[] lastName =["s","q","t","r","p"]; String[] salary =["40","20","50","30",10"]; String[] phoneNo= ["4","2","5","3","1"];
means for example if index of
"a"
fromfirstName
Changes from 0 to 4, respective index of"p"
,"10"
,"1"
to be changed from 0 to 4.. -
Admin about 8 yearsWhat if my Array is of X length....??
-
Grant Nilsson about 8 yearswell all of the examples you used above were using the same sorting order. so i assumed you had a set order and length. does the shuffle need to be random?
-
Admin about 8 yearsthats what i need for arrays of Unknown some X length
-
Grant Nilsson about 8 yearsif you need to randomize the list then see the comments above from others
-
Admin about 8 yearsthanks for ur answer but i have got solution for it