How to skip the first iteration in a for each loop?
Solution 1
You can:
- use a classic loop
- use a boolean to know if one iteration is the first
- with java 8 use a stream and skip 1
For example:
map.entrySet().skip(1).forEach(...);
Solution 2
Probably not elegant, but it will work and won't have any noticeable performance impact:
boolean isFirst = true;
for(...) {
if(!isFirst) {
// your code
} else {
isFirst = false;
}
}
Solution 3
Best to use an Iterator
:
final Map<String, String> map = new HashMap<>();
final Iterator<Entry<String, String>> iter = map.entrySet().iterator();
if (!iter.hasNext()) {
//map is empty - handle
}
iter.next();
while (iter.hasNext()) {
final Entry<String, String> e = iter.next();
//your code
}
I prefer it to the boolean
approach because you don't need to check the flag in every iteration. This approach also gives the the latitude to consume more than one entry in each iteration as long as you recheck hasNext()
before consuming each additional item.
P.S.
Please stick to Java naming conventions. Your class co
should be in PascalCase
. Also Co
is probably not the best name for a class
- try to pick meaningful names for everything from classes to variables.
Solution 4
Here are two basic options:
a) You could use a "for each" loop and set a temp boolean to help.
// set a boolean outside the loop that we query and update inside it
boolean firstRun = true;
for (Object obj : someList) {
if (firstRun) {
firstRun = false;
doFirstIterationOnly();
continue;
}
doExceptOnFirstIteration();
}
b) A "for i" loop where you start from the second element and ignore the first element entirely.
// start 'i' on index 1 instead of the usual index 0
for (int i=1; i < collection.size(); i++) {
Element neverFirst = collection.get(i);
doSkippingFirstElement();
}
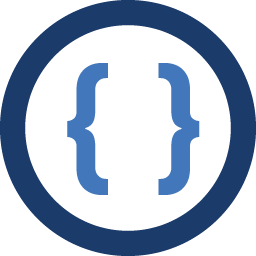
Admin
Updated on June 09, 2022Comments
-
Admin almost 2 years
Programming is new to me and i am trying to get my head around some of the concepts. I am trying to make a simple applet that displays the capital cities of Europe on a map on join them using the drawLine method. I have encountered a problem where i cannot successfully join the 2 capital cities together with a line. I think i understand why but i cannot think of a way to around it. The second two parameters in the draw method are the same as the first two, but i cannot make it skip the first iteration. This is all new to me and i am trying to learn from a book and the web at the moment.
public void paint(Graphics g) { super.paint(g); g.drawImage(image, 0, 0, this); for (Entry<String, co> entry : map.entrySet()) { g.setColor(Color.BLUE); g.fillOval(entry.getValue().a, entry.getValue().b, 5, 5); g.setColor(Color.BLUE); g.drawString(entry.getKey(), entry.getValue().a+7, entry.getValue().b+7); g.setColor(Color.RED); g.drawLine(entry.getValue().a, entry.getValue().b, 0, 0);//Problem } }
Can some one push me in the right direction? I was thinking of using an iterator instead of a for each loop, that is the only idea i have in my head at the moment.