How to sleep a C++ Boost Thread
Solution 1
Depending on your version of Boost:
Either...
#include <boost/chrono.hpp>
#include <boost/thread/thread.hpp>
boost::this_thread::sleep_for(boost::chrono::milliseconds(100));
Or...
#include <boost/date_time/posix_time/posix_time.hpp>
#include <boost/thread/thread.hpp>
boost::this_thread::sleep(boost::posix_time::milliseconds(100));
You can also use microseconds, seconds, minutes, hours and maybe some others, I'm not sure.
Solution 2
From another post, I learned boost::this_thread::sleep
is deprecated for Boost v1.5.3: http://www.boost.org/doc/libs/1_53_0/doc/html/thread/thread_management.html
Instead, try
void sleep_for(const chrono::duration<Rep, Period>& rel_time);
e.g.
boost::this_thread::sleep_for(boost::chrono::seconds(60));
Or maybe try
void sleep_until(const chrono::time_point<Clock, Duration>& abs_time);
I was using Boost v1.53 with the deprecated sleep
function, and it aperiodically crashed the program. When I changed calls to the sleep
function to calls to the sleep_for
function, the program stopped crashing.
Solution 3
firstly
boost::posix_time::seconds secTime(1);
boost::this_thread::sleep(secTime);
secondly
boost::this_thread::sleep(boost::posix_time::milliseconds(100));
Solution 4
I learned the hard way that at least in MS Visual Studio (tried 2013 and 2015) there is the huge difference between
boost::this_thread::sleep(boost::posix_time::microseconds(SmallIterval));
and
boost::this_thread::sleep_for(boost::chrono::microseconds(SmallIterval));
or
std::this_thread::sleep_for(std::chrono::microseconds(SmallIterval));
when interval is smaller than some rather substantial threshold (I saw threshold of 15000 microseconds = 15 milliseconds).
If SmallIterval is small, sleep() does instantaneous interruption. sleep(100 mks) behaves as sleep(0 mks).
But sleep_for() for the time interval smaller than a threshold pauses for the entire threshold. sleep_for(100 mks) behaves as sleep_for(15000 mks).
Behavior for intervals larger than threshold and for value 0 is the same.
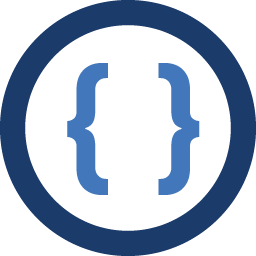
Admin
Updated on September 07, 2020Comments
-
Admin over 3 years
Seems impossible to sleep a thread using boost::thread. Method sleep requires a system_time but how can I build it?
Looking inside libraries doesn't really help much...
Basically I have a thread inside the function that I pass to this thread as entry point, I would like to call something like
boost::this_thread::sleep
or something, how to do this?
Thank you
-
Admin over 13 yearsYes, thank you... I didn't pay attention using this_thread but just boost::thread and nothing worked:) Thansk
-
Tim MB over 10 yearsThis gives me the following error (using Xcode 5 / LLVM): error: no viable conversion from 'boost::posix_time::microseconds' (aka 'subsecond_duration<boost::posix_time::time_duration, 1000000>') to 'const system_time' (aka 'const boost::posix_time::ptime')
-
kgbook over 5 yearsuse
boost::this_thread::sleep_for
instead ofboost::this_thread::sleep
, the latter is deprecated, see boost::this_thread::sleep.