How to solve TypeError: environment.teardown is not a function
Solution 1
You shouldn't need to install jest-cli yourself. It should come out of the box.
Try the following:
- Delete package-lock.json, yarn.lock and node_modules
- Remove jest from the dependencies in package.json
- Then do
npm install
oryarn install
.
Solution 2
Add a fresh version of "jest-environment-jsdom" to file "package.json".
Solution 3
If you have not used create-react-app
for the project:
- Just remove
jest, jest-cli, jest-environment-jsdom
frompackage.json
. - Delete folder node-modules, file package-lock.json and file yarn.lock.
- Use
npm --save i
oryarn add
to install all of them so that each of them have same version.
This should work!
In my case I was missing the jest-environment-jsdom
package.
Solution 4
In my case it throws this error because I create my React project using create-react-app
and install jest
. In certain way it conflicts.
I solve it by removing jest
from my project and using the create-react-app
test script.
Solution 5
After an evening of banging my head on this issue, I think I've finally resolved it. First, let me describe the problem:
I was also getting:
TypeError: environment.teardown is not a function
Because I was trying to upgrade Jest to the latest version. When starting an app with Create-React-App, it installs an earlier version of Jest that does not include all of the features. I was interested in using the inlineSnapshots feature, which allows you to assert rendered components against HTML markup inside your test file.
There are a few things not included in the documentation of either Create-React-App or Jest which you should be aware of. If you want to use newer Jest features, like inlineSnapshots, then you need to do the following:
- yarn adds a fresh version of Jest and jest-environment-jsdom
- yarn add prettier --dev (This is to facilitate rewriting back into the source file.)
- Add a Jest env docblock to the very top of each test script.
A docblock is a JavaScript block comment with a pragma mark "@". For setting the Jest environment, you would add this to the top of every test file:
/**
* @jest-environment jsdom
*/
This instructs jest to run the test in the jsdom environment, which should match what is passed in the test scripts section of your package.json file. Mine looks something like this (ignore the parts with react-app-rewired):
"scripts": {
"start": "yarn run flow && react-app-rewired start",
"build": "yarn run flow && react-app-rewired build",
"test": "react-scripts test --env=jsdom"
}
After doing all of this, I was finally able to get inlineSnapshots to work.
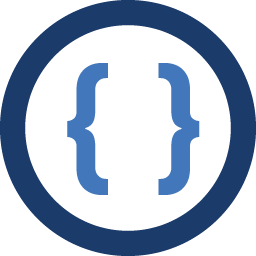
Admin
Updated on July 22, 2022Comments
-
Admin almost 2 years
I can't test an application which was created with create-react-app.
All guides says that test is working by default, but when I try "yarn test", it requires install 'jest-cli' and after installation gives an error:
TypeError: environment.teardown is not a function
-
Weijing Jay Lin almost 6 yearsthen how to test a single file? npm test?
-
MstrQKN almost 6 yearsNot really sure Weijing, but I think your answer should be here: github.com/facebook/create-react-app/blob/master/packages/…
-
Wilber Hinojosa almost 6 years@WeijingJayLin , jest test files based on patterns to match files. If you want to test a single file, you should specify an specific pattern for its file name.
yarn run test /specificfolder/otherspecific/file.spec.js
If that pattern only matches one file. Then you will test only a single file. the one you want! -
Ryan Walker over 5 yearsif you used
create-react-app
make sure"test": "react-scripts test --env=jsdom"
is your test script. -
Rucha Bhatt Joshi over 5 yearsHello,Try to avoid comment in answer unless you have "specific detail" answer. Please use comment box below and Once you have sufficient reputation you will be able to comment on any post. :)
-
pzrq over 4 yearsThis inspired me and worked,
npm i -D jest-environment-jsdom@24
-
Peter Mortensen over 3 years
module.export
? Notmodule.exports
?